Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial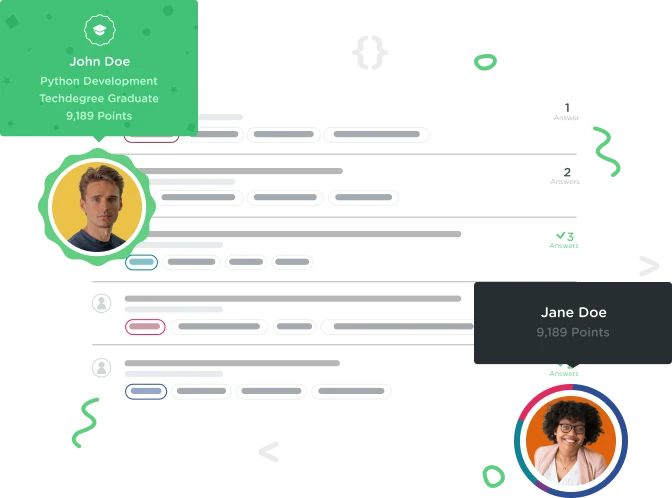
Errin E. Johnson
22,458 PointsIm not understanding how to check if number is an integer. Not sure if its a syntax or logic misunderstanding?
I used a try and except block to test for integer. I was thinking I could just say try: int(num) and if this is true then return ... but that didnt work. I tried saving it into a variable such as x = int(num), logic if x is an integer then return ...
Then I tried a conditional statement which is included in my final code submission. Im not sure what Im missing here
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(num):
try:
if num == int(num):
return num * num
except TypeError:
return len(num) * num
2 Answers
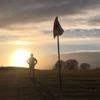
Stuart Wright
41,120 PointsIf you pass a float, say 3.5 to that function, nothing will be returned.
It will fail the condition so num * num will not be returned, but there also won't be an error, so the except block won't be executed.
Here is one correct solution:
def squared(num):
try:
num = int(num)
return num * num
except ValueError:
return len(num) * num
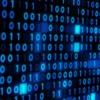
Alexander Davison
65,469 PointsYou have a logical error, not a syntactical error.
This seems to pass:
def squared(num):
try:
return int(num) * int(num)
except ValueError:
return num * len(num)
- You should catch the
ValueError
error, not theTypeError
error. - Multiplying a string by a number makes more sense then multiplying a number by a string. You don't have to change this, though.
- You should return
int(num) * int(num)
, because ifnum
was the string'1'
, you would have to convert it to an integer before adding. Just callingint(num)
won't actually change the value ofnum
!
I hope this helps
Happy coding!
~Alex
Errin E. Johnson
22,458 PointsThank You, I need to read up on the except errors: :)
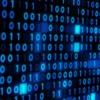
Alexander Davison
65,469 PointsTo see which error a try
block could cause, try doing something that the try
block might do that will cause an error.
For this situation, I knew that it was a ValueError
because I tested this (which is what might happen with our try
block):
>>> int('a')
...
ValueError: Invalid literal for base 10: 'a'
Errin E. Johnson
22,458 PointsErrin E. Johnson
22,458 PointsThank You, have another question. num = int(num) ; would it matter if num was called x; therefore: x = int(num)? or is num the argument understood as being the same when declared in a variable. variable x then wouldn't make sense, you couldn't give variable any name. Trying to understand how python complies and interprets this code block, what does it "see" line by line. ? The above code work correct , plus I had to change the except error to ValueError:
Stuart Wright
41,120 PointsStuart Wright
41,120 Pointsx = int(num) would be fine too. Then you would need to return x * x. There is nothing special about 'num' - it's just the variable name in this exercise. Integers are immutable so cannot be changed 'in place' . To convert to integer, it needs to be assigned to another variable. Reusing the same variable (as I've done in my example), or using another name such as x are both fine.