Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial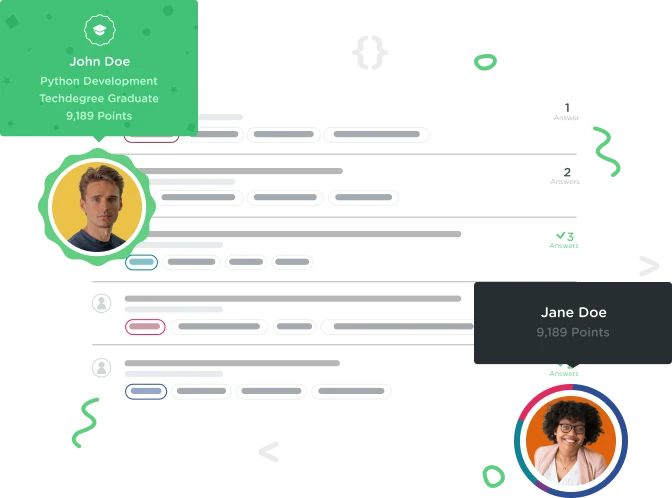

ashleytan
6,131 PointsI'm not sure what I'm missing (TypeError: from_string() missing 1 required positional argument)
I'm not entirely clear on what I'm supposed to do and I'm not sure what the error means either - TypeError: from_string() missing 1 required positional argument
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
def from_string(self, spoken_morse):
spoken_morse = spoken_morse.split["-"]
written_morse = []
for blip in spoken_morse:
if blip == "dash":
written_morse.append("_")
elif blip == "dot":
written_morse.append(".")
self.pattern = written_morse
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
2 Answers

Kip Yin
4,847 PointsSo here you need to define a classmethod
called from_string
, which returns an instance of the class Letter
.
Firstly, your from_string()
is not a classmethod
. Simply wrap the function with@classmethod
and change self
to cls
:
@classmethod
def from_string(cls, spoken_morse):
# the rest of the code
Secondly, this class method needs to return an instance of Letter
with written_morse
as its argument:
@classmethod
def from_string(cls, spoken_morse):
# main logic
return cls(written_morse) # instead of `self.pattern = written_morse`.
Lastly, I see that you used square brackets with the .split
method. It's probably a typo, but you should use parentheses instead:
...
def from_string(cls, spoken_morse):
spoken_morse = spoken_morse.split("-") # instead of .split["-"]
...
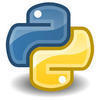
Gerald Wells
12,763 PointsIt appears as though you didn't watch the video previous to the challenge... you should probably go back through it; your syntax for @classmethod
is careless.
Here is the answer but I am not really helping you with the solution. I purposely made an obscure solution so hopefully you will go back and take the time to comprehend Kenneth Love's video.
@classmethod
def from_string(cls, string):
char = {"dash": "_", "dot": "."}
pattern=[c for i in list(map(lambda k: char[k], string.split("-"))) for c in i]
return cls(pattern)

ashleytan
6,131 PointsI didn't realise I completely missed a video! I went back to watch it and now everything is clear. Thanks.
Gerald Wells
12,763 PointsGerald Wells
12,763 Pointsits an undersore not a hyphen
Kip Yin
4,847 PointsKip Yin
4,847 PointsWhat is?
Gerald Wells
12,763 PointsGerald Wells
12,763 PointsLook at the challenge
Kip Yin
4,847 PointsKip Yin
4,847 PointsI did. I don't understand what "it" is referring to.
Kip Yin
4,847 PointsKip Yin
4,847 PointsYou mean
.split("-")
?Gerald Wells
12,763 PointsGerald Wells
12,763 PointsI misread your code, I am a moron. disreguard
ashleytan
6,131 Pointsashleytan
6,131 PointsThank you for the detailed and clear response!