Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial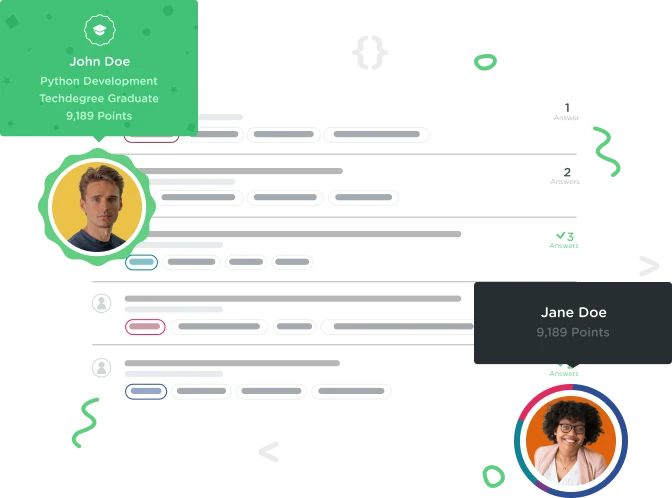

Bill Tihen
17,790 PointsI'm getting a swift comile message to check preview for the compile errors - when I click on preview the page is blank a
let value = 200 let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15 let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below let result = value % divisor
if result == 0 { let isPerfectMultiple = true } else { let isPerfectMultiple = false }
what is the issue?
// Enter your code below
let value = 200
let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15
let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below
let result = value % divisor
if result == 0 {
let isPerfectMultiple = true
} else {
let isPerfectMultiple = false
}
3 Answers

Nichlas Kondrup
17,748 PointsYou don't have use an if-else statement. You can simply say that the calculation should return 0
// Enter your code below
let value = 200
let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15
let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below
let result = value % divisor
let isPerfectMultiple = value % divisor == 0

Bill Tihen
17,790 PointsOK thanks - that works. But why do I get a compile error on my code (without any error)? I don't get a compile error in xcode nor in ibm's swiftlang site

Nichlas Kondrup
17,748 PointsI guess it's because you have two different constants both named isPerfectMultiple. If you want to use an if-else statement you can write like this:
// Enter your code below
let value = 200
let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15
let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below
let result = value % divisor
let isPerfectMultiple: Bool
if result == 0 {
isPerfectMultiple = true
} else {
isPerfectMultiple = false
}

Bill Tihen
17,790 PointsAh cool - that is what I was overlooking.