Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial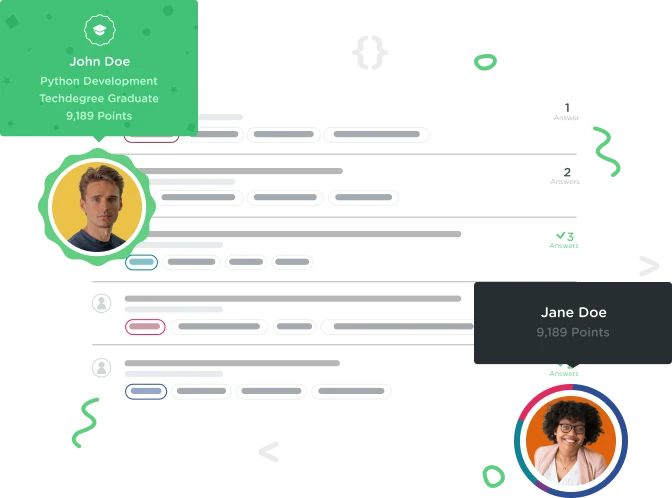

Thomas Williams
9,447 PointsIllegal start of 'for'??
package com.example;
import java.util.List; import java.util.Set; import java.util.TreeSet;
public class Blog { List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() { return mPosts; } Set<String> getAllAuthors = new TreeSet<String>(); for (BlogPost post : Blog) { getAllAuthors.addAll(post.getAuthor); }
}
I'm not sure whats wrong with this but i'm getting illegal start of the 'for' loop?? Please help!
3 Answers

James Simshaw
28,738 PointsHello,
You want getAllAuthors to be a method of the Blog class. Then inside that method, you want to do all of the logic for creating the set, adding all of the authors to it, and return the set.

James Simshaw
28,738 PointsHello,
It looks like some of your code is not inside any functions.
Set getAllAuthors = new TreeSet(); for (BlogPost post : Blog) { getAllAuthors.addAll(post.getAuthor); }
is the code I'm referring to. It looks like some of it might have gotten mistakenly deleted.

Thomas Williams
9,447 PointsHaha, that is the only bit of code I have written, the only bit of code missing is String after Set and TreeSet, it hasn't pasted for some reason. The challenge was to add a method to this Blog class that takes the author from each blogpost and puts them into an alphabetically ordered list using the java.util.Set interface. The getAuthor method is in the BlogPost class. To my mind this code is saying create a new alphabetically ordered list called getAllAuthors, then for each blogpost in Blog (a list of blogposts) get the author and add it to the getAllAuthors list, hence, completing the challenge. Clearly my understanding isn't quite what it should be yet!
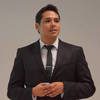
Juan Santiago
3,766 PointsThis is my code, I'm stuck with the compiler errors (I've tried a lot of combinations with the "for" statement and the .getAuthor variable) but I can't figure it out why :/ HELP!
public Set<String> getAllAuthors() { Set<String> allAuthors = new TreeSet<String>(); for (Blog post:posts) { allAuthors.add(post.getAuthor()); } return allAuthors; }
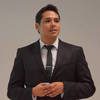
Juan Santiago
3,766 PointsI solve it :3
public Set<String> getAllAuthors() {
Set<String> allAuthors = new TreeSet<String>();
for (BlogPost post: mPosts) {
allAuthors.add(post.getAuthor());
}
return allAuthors;
}
yok558
Courses Plus Student 3,627 Pointsyok558
Courses Plus Student 3,627 PointsDid you complete this challenge? I've stuck with it.
public Set<String> getAllAuthors() { Set<String> allAuthors = new TreeSet<String>(); for (??? ???: ???) { allAuthors.addAll(???.getAuthor()); } return allAuthors;
Need something like that?
James Simshaw
28,738 PointsJames Simshaw
28,738 PointsIts close, you need to make sure you define what the sets are holding like
Set<SomeType> someSet = new TreeSet<>();
as well as in your returntype. For the for loop, consider what you're looping over, you need to define it as
for(Type variable: someList)
yok558
Courses Plus Student 3,627 Pointsyok558
Courses Plus Student 3,627 PointsGot this:
But an error occured:
James Simshaw
28,738 PointsJames Simshaw
28,738 PointsFirst, the posts are in the member variable mPosts. Second, you might want to use Set.add instead of Set.addAll since you're only adding a single item and not a collection.
yok558
Courses Plus Student 3,627 Pointsyok558
Courses Plus Student 3,627 PointsI did it, man! Thank you very much!