Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial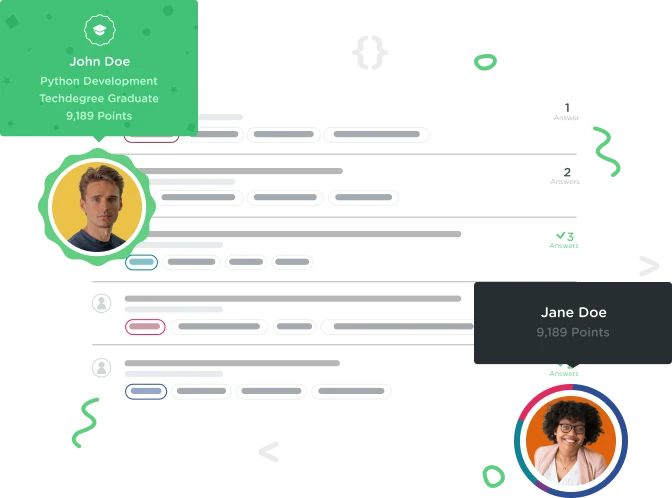
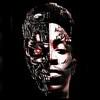
Tinashe chikamba
1,657 Pointsillegal arguments
Protect the call to kart.drive by handling the IllegalArgumentException that is thrown when not enough battery remains. Print out the message from the exception to the screen as you catch the exception.
4 Answers
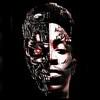
Tinashe chikamba
1,657 Pointsthanks it really helped
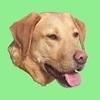
alastair cooper
30,617 Pointssorry, the call to drive should be included in the try block. Like this...
public static void main(String[] args) {
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(42);
} catch(IllegalArgumentException iae){
System.out.println(iae.getMessage());
}
}
If this doesn't work, follow this link...
hope this helps
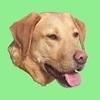
alastair cooper
30,617 Pointsyou have a catch block, but no try block before it.
Try this...
public static void main(String[] args) {
try{
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
kart.drive(42);
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage());
}
}
hope this helps
PS to make code snippets appear like this (in a black console screen), see the link 'Markdown Cheatsheet' below
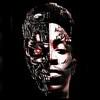
Tinashe chikamba
1,657 Points./Example.java:9: error: illegal start of type } catch (IllegalArgumentException iae) { ^ 1 error
kart.drive(42); } catch (IllegalArgumentException iae) { System.out.println(iae.getMessage()); } } so i tryed the code u gave but its still giving me this error
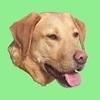
alastair cooper
30,617 PointsYou need to surround it with a try catch block. In the catch block you can print out the exception message.
Show me what you have tried and I'll help you fix it
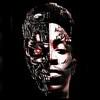
Tinashe chikamba
1,657 Pointsthanks it worked
Tinashe chikamba
1,657 PointsTinashe chikamba
1,657 Pointsclass Example {
public static void main(String[] args) { GoKart kart = new GoKart("purple"); if (kart.isBatteryEmpty()) { System.out.println("The battery is empty"); } kart.drive(42); } catch (IllegalArgumentException iae) { System.out.println(iae.getMessage()); } }
what am i missing