Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial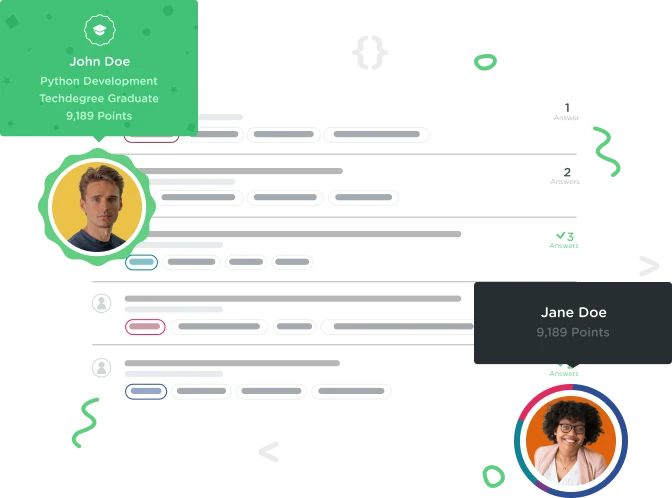
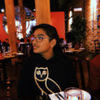
Haardik Gupta
Full Stack JavaScript Techdegree Student 5,459 Pointsif else
i don't understand what i'm doing wrong
i'm being asked to
Inside the if statement, return a string equal to the value of the name property followed by the string " is now playing!". Use bracket notation.
const player1 = {
name: 'Ashley',
color: 'purple',
isTurn: true,
play: function(){
// write code here.
if (this.isTurn) {
console.log(this.name.value + 'is now playing');
}
}
}
4 Answers
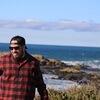
Fabio Faria
2,153 Pointsreturn player1['name'] + "string";

Zimri Leijen
11,835 PointsThere's several terms in the question you seem to have misunderstood.
return
is a keyword that causes a function to return
something.
Certain functions have an output, which is called the return
. One example would be a sum function like
function sum(a, b) {
return a + b;
}
const answer = sum(3, 2);
console.log(answer); // should log 5
Bracket notation, as apposed to dot notation is just a different way to access a value.
this.name
would be dot notation, while
this["name"]
would be bracket notation.
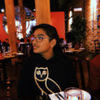
Haardik Gupta
Full Stack JavaScript Techdegree Student 5,459 Pointsi tried again my new code was
const player1 = {
name: 'Ashley',
color: 'purple',
isTurn: true,
play: function(){
// write code here.
if (this.isTurn) {
return (this[name] "is now playing);
}
}
}
but i got the error invalid or unexpected token

Zimri Leijen
11,835 Points const player1 = {
name: 'Ashley',
color: 'purple',
isTurn: true,
play: function(){
// write code here.
if (this.isTurn) {
return (this[name] "is now playing);
}
}
}
That's already quite good, note that return
doesn't need parenthesis, so you can just for example
return "some string"
Since you want to return a value and a string, you can either append the string to the value like this:
return value + "string"
or use string interpolation (template literal)
return `${value} string`
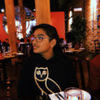
Haardik Gupta
Full Stack JavaScript Techdegree Student 5,459 Pointsthank you for the help
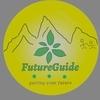
Sezare Miguel
9,229 Points``` const player1 = { name: 'Ashley', color: 'purple', isTurn: true, play: function(){ // write code here. if (this.isTurn) { return Ashely + "is now playing"; } } }