Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial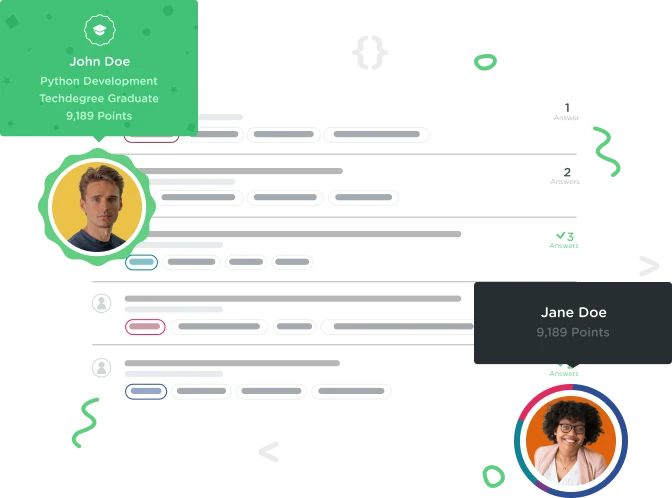

Gabriel Murut
5,418 PointsIDE - Finishin Treestory excercise
Hi everyone, I juste finished this exercise after a few hours coding, and I had a lot of errors. At the end it worked.
I have seen there was a while since the last question, and some of them are unanswred.
Please feel free to contact me if you need any assistance on this!
I'm leaving a copy of the code if it helps.
Main
package com.teamtreehouse;
import java.io.IOException; import java.util.Arrays; import java.util.List;
public class Main {
public static void main(String[] args) {
// write your code here
Prompter storyPrompter = new Prompter();
String story = null;
try {
story = storyPrompter.promptForStory();
} catch (IOException e) {
e.printStackTrace();
}
Template tmpl = new Template(story);
storyPrompter.run(tmpl);
}
}
Prompter
package com.teamtreehouse;
import java.io.; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.;
public class Prompter { private BufferedReader mReader; private Set<String> mCensoredWords; private String story;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
String storyResults = tmpl.render(results);
System.out.printf("Your TreeStory:%n%n%s", storyResults);
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException{
// TODO:csd - Prompt the user for the response to the phrase, make sure the word is censored, loop until you get a good response.
String wordStory = null;
boolean validation = false;
do {
System.out.printf("Please fill in %s : ", phrase);
wordStory = mReader.readLine();
if (mCensoredWords.contains(wordStory.toLowerCase())) {
validation = true;
System.out.printf("We do not accept that languagge %n");
} else validation = false;
}
while (validation);
return wordStory;
}
public String promptForStory() throws IOException {
System.out.printf("Please introduce your TreeStory %n" + "Do not forget double underscore for the words to fill %n"+ ":");
String treeStory = mReader.readLine();
return treeStory;
}
public String getStory() {
return story;
}
public void setStory(String story) {
this.story = story;
}
}