Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial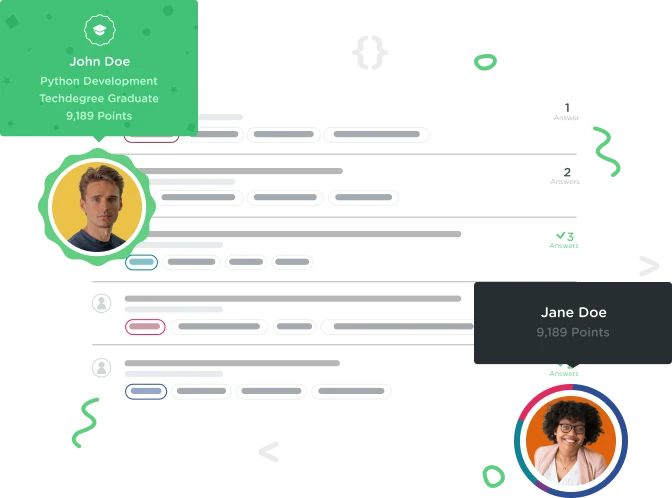
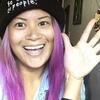
Jahnel Madarang
Full Stack JavaScript Techdegree Student 6,798 PointsI wrote two functions. Why is + string to number required for my function to run properly but not in the other?
Hello!
I wrote two functions for this challenge. While testing, the first function works just fine. However, my second function was giving me numbers way higher than the upper number given until I put the (+) in front of the variables to convert from string to text. I'm fine doing this but am confused as to why the first function runs properly without needing the (+). Looking forward to getting help understanding all this, thank you!
1:
function getRandomNumber(lower, upper) {
const number = Math.floor(Math.random() * (upper - lower + 1)) + lower;
return document.querySelector('main').innerHTML = `Your random number between ${lower} and ${upper} is ${number}!`;
}
getRandomNumber( 5, 20 );
2:
function getRandomNumber() {
const lower = prompt("What is a number less than 10?");
const upper = prompt("What is a number greater than 10?");
const number = Math.floor(Math.random() * (+upper - +lower + 1)) + +lower;
return document.querySelector('main').innerHTML = `Your random number between ${lower} and ${upper} is ${number}!`;
}
getRandomNumber();

Abdulsalam Jumah
3,679 Pointsjust to add to Robert's comment if I may you can get in the habit of testing things out and checking by using typeof and console.log to make sure of the values you are getting which is even more helpful when using conditional statements.
4 Answers
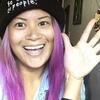
Jahnel Madarang
Full Stack JavaScript Techdegree Student 6,798 PointsAh yes, makes complete sense. Thank you so much for explaining!! :)

Jason Cheung
3,227 Pointsfunction getRandomNumber(lower=1, upper=100) {
const randomNumber = Math.floor(Math.random() * (upper - lower + 1) ) + lower;
return randomNumber;
}
const lower = prompt("Enter the minimum number");
const upper = prompt("enter the maximum number");
if (Number.isInteger(+lower)===true){
if (Number.isInteger(+upper) === true){
alert(`${getRandomNumber(parseInt(lower), parseInt(upper))} is the random number between ${lower} and ${upper}`)
}else{
alert("Please enter numeric numbers")
}
}else{
alert("Please enter numeric numbers")
}
I have done much more than what it requested, but I think this is more interactive and can be an more user-friendly generator

Abdulsalam Jumah
3,679 PointsYou could have used the and (&&) operator instead of using two if statements.. which even leads to just one else block:
function getRandomNumber(lower=1, upper=100) {
const randomNumber = Math.floor(Math.random() * (upper - lower + 1) ) + lower;
return randomNumber;
}
const lower = prompt("Enter the minimum number");
const upper = prompt("enter the maximum number");
if (Number.isInteger(+lower)===true && Number.isInteger(+upper) === true) {
alert(`${getRandomNumber(parseInt(lower), parseInt(upper))} is the random number between ${lower} and ${upper}`)
} else {
alert("Please enter numeric numbers")
}

Jason Cheung
3,227 Pointsah yea thanks for the great recommendation, didnt realize that existed when i code, thanks a lot for the reply

Kashyap Sodha
Courses Plus Student 5,686 PointsAny input taken from the user is considered as a 'string' by JavaScript. For the performing operations, we need to convert the input (lower and upper in this case) to an integer or a float (a number essentially). We can do so by using parseInt(), parseFloat() functions. Example: let lower = prompt("Enter the minimum number"); lower = parseInt(lower);
Robert Gulley
Front End Web Development Techdegree Student 10,722 PointsRobert Gulley
Front End Web Development Techdegree Student 10,722 PointsHi Jahnel! -
In your first set of code, you are passing 2 values (5 & 20) to the function without quotes around them. JavaScript interprets these as numbers since they don't have quotes around them. Since they are passed as numbers into your function, JavaScript is able to do the math with the upper and lower variables in your function.
In your second set of code, you are prompting the user for numbers. When you prompt a user in this manner, regardless of whether the item they type is a number or not, JavaScript is always going to interpret it as a string. Since JavaScript thinks that upper and lower are strings, it doesn't know how to add 1 to them. For example, you can't say apple + orange + 1. Adding the + in front of these variables lets JavaScript know that they are numbers and not strings, and once JavaScript knows they are numbers, it knows how to add those.
As an example, say that your user entered 8 for the first prompt (lower), and 15 for the second prompt (higher). Without putting the + in front of upper or lower, JavaScript thinks these values are '8' and '15', not 8 and 15.
I hope this helps!