Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial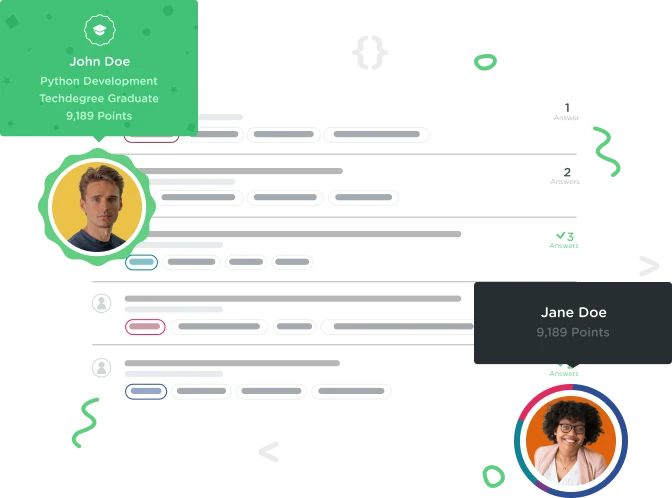
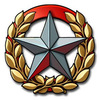
Matt Malone
11,437 PointsI took out all of the TODOs, but it still says that I've left the TODOs in the code.
If you could tell my the problem with the TODOs, I'd appreciate it.
// After you've completed the TODOs locally paste Main.java here
package com.teamtreehouse;
import java.util.Arrays;
import java.util.List;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = prompter.promptForStoryTemlate();
Template tmpl = new Template(story);
prompter.run(tmpl);
}
}
// After you've completed the TODOs locally paste Prompter.java here
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.Scanner;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
public String promptForStoryTemlate() {
Scanner reader = new Scanner(System.in); // Reading from System.in
System.out.println("Enter a story template: ");
String sentence = reader.nextLine();
return sentence;
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public String run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
// System.out.println(tmpl.render(results));
System.out.printf("Your TreeStory:%n%n%s", tmpl.render(results));
return null;
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) {
Scanner reader = new Scanner(System.in); // Reading from System.in
System.out.println("Enter a word: ");
String word = reader.next();
return word;
}
}
2 Answers
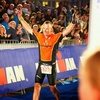
Steve Hunter
57,712 PointsHi Andrew,
The first line of both those files contains the string TODO
- remove those!
The TODO
checker is just looking for that string within the files, not just at the point you need to do something. So just remove those characters and you'll get a proper analysis of your code.
Let me know how you get on.
Steve.
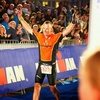
Steve Hunter
57,712 PointsOK - you've done this in an IDE so you've used Scanner
. That's fine but the challenge needs you to cover off the potential of the reader.nextLine()
coming back with nothing.
I surround this within a rudimentary test to see if hasNext()
is true
. Something like:
String sentence = "";
if (reader.hasNext()){
sentence = reader.nextLine();
}
return sentence;
I've covered off the String
being declared outside the if
as well.
The other way round this is to use the Console
object that the challenge lets you use. Then you can simply use readLine()
, which I know can be challenging within the IDE environment!
I've enclosed all you usages of nextLine()
within an if
and I now get real problems, i.e. ones related to the callenge in question. Pseudo tests 1 & 2 are failing. That's good - at least the syntax is fine!
Shout me if you need a hand.
Steve.
Matt Malone
11,437 PointsMatt Malone
11,437 PointsOkay, I'll try that out. It looks like I've got some other problems with it as well. Thanks.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsThat's good - changing error message means you're making progress. Let me know what the new messages are and I'll help you fix them.
Steve.