Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial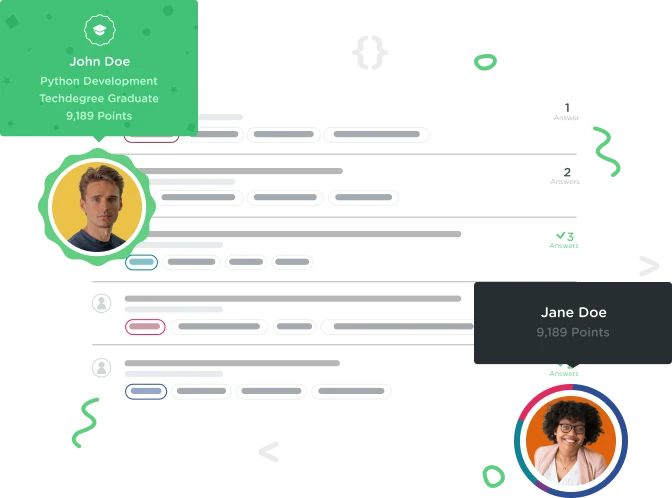
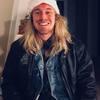
kristianemil
4,632 PointsI think I have it, but the BREAK won't seem to work
Not the pretties nor the most efficient way......say it works. But it doesn't!
def disemvowel(word):
word = []
word.extend(word.lower())
try:
word.remove("a")
except ValueError:
try:
word.remove("e")
except ValueError:
try:
word.remove("i")
except ValueError:
try:
word.remove("o")
except ValueError:
try:
word.remove("u")
except ValueError:
break
return word
2 Answers

Christian Mangeng
15,970 PointsHere's what I mean (the solution). I guess it will make some things clearer for you. But you really should check out the documentation about for loops. They are extremely important and are used frequently.
def disemvowel(word):
vowel = ("a", "e", "i", "o", "u")
for letter in word:
if letter.lower() in vowel:
word = word.replace(letter, "")
return word
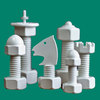
Steven Parker
231,128 PointsYou don't have a loop.
So I'm not sure what you'd be expecting break to do. Also, you might need a different strategy if you want to remove more than one vowel from a word. Don't forget to consider that some words may even have more than one of the same vowel.
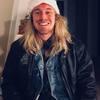
kristianemil
4,632 PointsAlright, besides the BREAK. Shouldn't this function sorta like a loop? I mean: try this; until the error message, then try the next thing and so on?
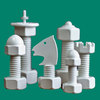
Steven Parker
231,128 PointsWell, yes, you might want to have a loop. I was just pointing out that you don't have one currently.

Christian Mangeng
15,970 PointsBreak does exactly the opposite of that: it breaks out of the loop. So break requires to be in a loop first. It is the "while" statement that can initialize a loop that continues as long as a certain condition is fulfilled (or endlessly). Also, break is not a function.
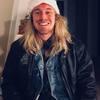
kristianemil
4,632 PointsSure thing Steven. I've been at it for hours now and stared blind on my code. Imma try figure that loop doop out!
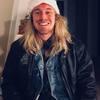
kristianemil
4,632 Pointssoo... a while loop kinda like this?
def disemvowel(word):
word = []
word.extend(word.lower())
while word:
try:
word.remove("a")
except ValueError:
continue
try:
word.remove("e")
except ValueError:
continue
try:
word.remove("i")
except ValueError:
continue
try:
word.remove("o")
except ValueError:
continue
try:
word.remove("u")
except ValueError:
return word

Christian Mangeng
15,970 PointsThe problem is that you need a better way to solve this task. The best way to solve it may be to use a "for" loop and iterating over the single characters of the word, then ask if this letter lowercased is contained in the list of lowercased vowels. If it is, you remove it. I would recommend using the replace() method.
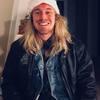
kristianemil
4,632 PointsThis is what my head is thinking:
get word
remove ("a", "e", "i", "o", "u") from word.lower()
remove ("A", "E", "I", "O", "U") from word.upper()
return word
I know its not Python or any other language for that matter, but it's sorta what I want.
I don't know where to fit that loop?

Christian Mangeng
15,970 PointsTry using this in your code. You will be able to do the rest. Take your time to overthink it.
for letter in word:
if letter.lower() in ("a", "e", "i", "o", "u"):
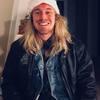
kristianemil
4,632 PointsThis is absolutely ridiculous, I mean I appreciate your help Christian(!), but this makes no sense to me what-so-ever, even though I feel this should be simple as pie.
Imma have to take this Python Basic Course from the top!

Christian Mangeng
15,970 PointsIt may be best for you to review some of the videos, or maybe check the documentation about "for loops". What this code basically does is what i wrote in that earlier comment. You can now use word.replace(letter, "") to replace the letter (if it is a vowel, which is being checked with that "for" loop) with an empty placeheolder (i.e. you remove it).
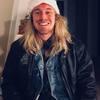
kristianemil
4,632 PointsBummer, still no luck. Can I ask where the word "letter" came to be? Should it be defined somewher?
def disemvowel(word):
for letter in word:
if letter.lower() in ("a", "e", "i", "o", "u"):
word.replace(letter, "")
if letter.upper() in ("A", "E", "I", "O", "U"):
word.replace(letter, "")
return word

Christian Mangeng
15,970 PointsIt could have any name you want. It is the name you give an element of the string (or list), in this case a letter of the word string (see for loops).
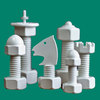
Steven Parker
231,128 PointsYou're getting really close.
But ... the replace method doesn't alter the original string. It returns a new string with the alterations. So you need to save the altered version.
Also, since you convert the letters to lowercase to compare with the vowels, you won't need to do it again with uppercase so you can save some code lines.
I'll bet you will get it now.
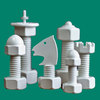
Steven Parker
231,128 Points Oh, darn. I really thought you were going to get it yourself using the hints in this thread. You were nearly there!
kristianemil
4,632 Pointskristianemil
4,632 PointsThanks man, I really appreciate you taking your time to help me!
Now I just gotta catch up on the whole loopy thingy, I found the official Python documentation for for-loops and while-loops – some good night reading right there!
Man I felt so good about this Python course until this challenge showed up. Back to the drawing board.
Catch you later ;-)
kristianemil
4,632 Pointskristianemil
4,632 PointsRight again brother. One thing at a time!