Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial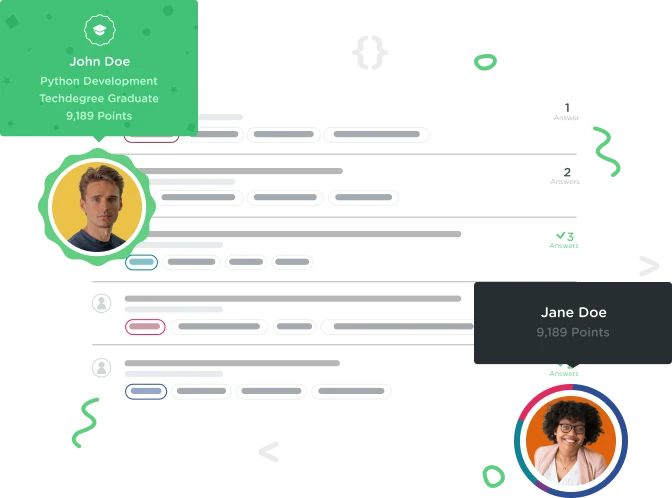

David Cruz
iOS Development Techdegree Student 1,818 PointsI need help with this code challenge
I don't know how to do it in the right way
struct Person {
let firstName: String
let lastName: String
func fullName(firstName first:String, lastName last:String) -> [Person] {
var result = [Person] ()
var name = firstName + "" + lastName
result.append(name)
return result
}
}
1 Answer
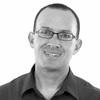
David Papandrew
8,386 PointsHi David,
The challenge wants you to add an instance method to the "Person" struct.
Here's the correct code:
struct Person {
let firstName: String
let lastName: String
func fullName() -> String {
return firstName + " " + lastName
}
}
//This is the 2nd part of the challenge, but I'm including it to show you how you call the method
let aPerson = Person(firstName: "David", lastName: "Cruz")
let myFullName = aPerson.fullName()
firstName and lastName will be passed as arguments when the struct is instantiated (in the example above, you can see this on the "let aPerson..." declaration.
Since these properties are being set, the "fullName" method doesn't need any parameters. All it needs to do is return a string.
Now when you get to step 2 of the challenge, you will create a Person instance and pass the first/last name values of your choosing. Then you can call the fullName method on the aPerson object to get the concatenated fullName.
Hope that helps.