Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial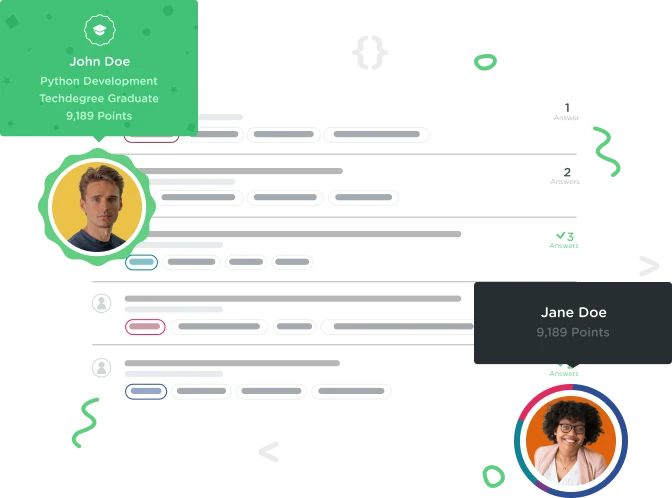
Miguel Angel Rodriguez
11,280 PointsI need help with the last code challenge.
The code in QuickFix.java
... java import com.example.model.Course; import com.example.model.Video;
import java.util.Map; import java.util.TreeMap; import java.util.List; import java.util.ArrayList; import java.util.Arrays;
public class QuickFix {
public void addForgottenVideo(Course course) { // TODO(1): Create a new video called "The Beginning Bits" Video newVideo = new Video("The Beginning Bits"); // TODO(2): Add the newly created video to the course videos as the second video. course.getVideos().add(1, newVideo); }
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) { Map<String, Video> byTitle = new TreeMap<String, Video>(); ArrayList<Video> courseList = new ArrayList<Video>(); courseList.add(course); for (Video video : courseList) { byTitle.put(video.getName(), video); } return byTitle; }
} ...
3 Answers

Sam Wilskey
3,420 PointsI believe the error is in the videosByTitle() method. First you are correct to create the Map as type TreeMap and there is nothing wrong with that.
Second I believe that you are attempting to make it more complicated then it needs to be. There is no reason to create an ArrayList to hold the Course. You are being passed only 1 course and so creating a ListArray of Courses is not needed. Even if you do want to continue using an ArrayList you created it with type Video and attempting to pass a Course in instead of a video. Just something to be aware of in the future.
Next take a look at the for loop:
for (Video video : courseList) {
byTitle.put(video.getTitle(), video);
}
this is almost correct but since we do not need the ArrayList since we already have access to the Course.getVideos method which returns a List we can change this to:
for (Video video : course.getVideos()) {
byTitle.put(video.getTitle(), video);
}
That should fix up the problems that you are having.
If you need anymore explanation please ask!
Hope this helps!
Miguel Angel Rodriguez
11,280 PointsThanks man, :) Works perfect!
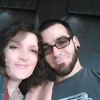
eric Crawford
2,381 PointsSo i know this an old post but if anyone reads this please explain. How is it that we store a list in an object of Video class which only has String? I understand that course is a List<Video>, but still not getting why we use
for (Video video : course.getVideos())
Sorry for my idiocracy
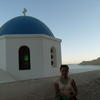
coryvancouver
1,670 PointsI keep getting an error in Task 2 that says: "Expected map to contain 0 keys but it contained 23". Shouldn't the map contain 23 keys since there are 23 videos?
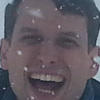
nihouse
7,160 PointsI guess so.
Maybe you should post all or some of your code -> it might help to find your mistake
Miguel Angel Rodriguez
11,280 PointsMiguel Angel Rodriguez
11,280 Points