Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial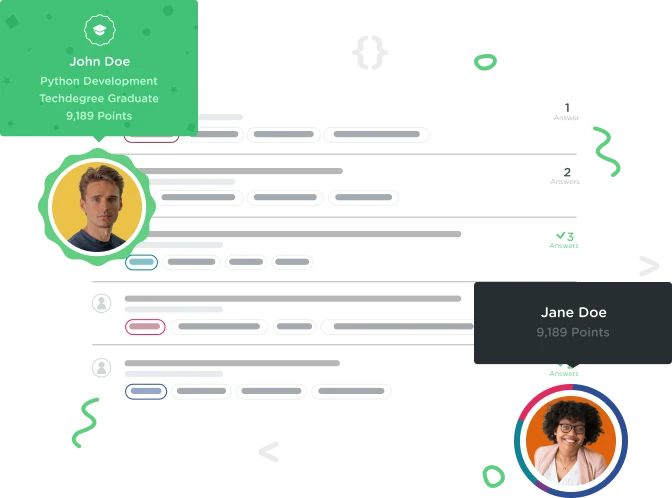

luisgomez7
5,557 PointsI need help understanding the function from https://teamtreehouse.com/library/improve-the-quiz-one-solution.
Please explain to me how the function in this program works. I understand it's using arrays as parameters but I DON'T understand how it knows which arrays to use given that they're not referenced anywhere in the function and why a for loop is required.
const correct = [];
const incorrect = [];
let correctAnswers = 0;
for( let i = 0; i < quiz.length; i++ ) {
let question = quiz[i][0];
let answer = quiz[i][1];
let response = prompt(question);
if( response.toLowerCase() === answer ) {
correctAnswers++;
correct.push(question);
} else {
incorrect.push(question);
}
}
function createListItems(arr) {
let items = '';
for (let i = 0; i < arr.length; i++) {
items += `<li>${arr[i]}</li>`;
}
return items;
}
let html = `
<h1>You got ${correctAnswers} question(s) correct</h1>
<h2>You got these questions right:</h2>
<ol>${ createListItems(correct) }</ol>
<h2>You got these questions wrong:</h2>
<ol>${ createListItems(incorrect) }</ol>
`;
document.querySelector('main').innerHTML = html;
5 Answers
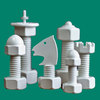
Steven Parker
241,957 PointsThe array passed as the argument is referenced in the function twice, both to control the loop (arr.length
) and within the loop to display each item (${arr[i]}
).
The purpose of the loop is to step through the array and cause each item to be displayed in a separate list item.

luisgomez7
5,557 PointsOk but what I don't understand is how the function knows that "arr" references the arrays. I've tried substituting all kinds of parameter names and everything works. Is it because the length property is associated with "arr" in the for loop? If so, how does the function know there's more than one array?

luisgomez7
5,557 PointsOk, I think I understand now. Somehow I didn't notice that the code below the function called the function twice, each time referring to a different array in the preceding lines of code. Once again, thank you for your help.

Margaret Martin
2,692 PointsI still don't understand. We have 3 different arrays in this code: the original array with the quiz questions, and then we make the incorrect answers and correct answers arrays. Not any of those are referenced in the function so how does the function know which array to pull the information from? What am I missing?
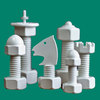
Steven Parker
241,957 PointsWhen the function is called, the array it will use is passed to it as the argument. Internally, the function references the array using the parameter name arr.
<ol>${ createListItems(correct) }</ol>
this time "arr" will be a reference to "correct"
<ol>${ createListItems(incorrect) }</ol>
this time "arr" will be a reference to "incorrect"

Margaret Martin
2,692 PointsOhhhhhhh, I see! It's because of what we are telling it to write to the inner.HTML! Now I feel dumb, hahaha. Thank you!
Steven Parker
241,957 PointsSteven Parker
241,957 PointsIn any function, parameters act only as placeholders for the arguments that will be provided when the function is called. The names of the parameters are not important other than they must be used consistently within the function.
The function doesn't "know" that the argument will be an array, it is written to require one. The later code could pass something other than an array, but if it did there would be a run-time error.
Each time the function is called, it processes only one array. It has no idea how many arrays there are or how many times it will be called.