Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial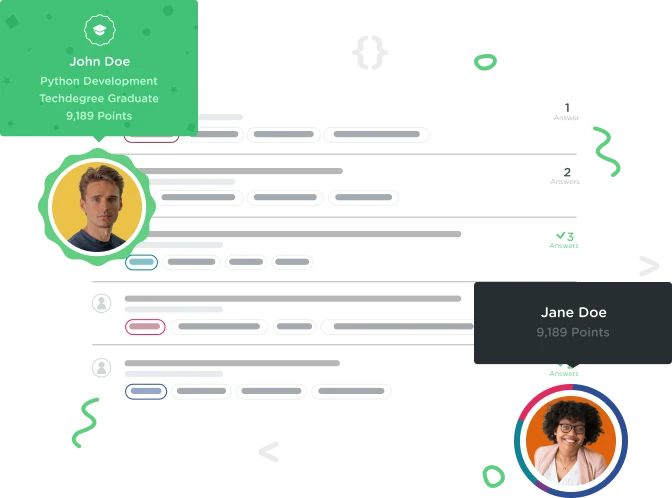

Manik Mehta
Courses Plus Student 2,729 Pointsi need help making my practice app.
This app returns a random result of a one or two dice roll:
//
// ViewController.swift
// RollMyDice
//
// Created by Manik Mehta on 8/21/17.
// Copyright © 2017 Manik Mehta. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var diceResult: UILabel!
let onediceResult = oneDice()
let twodiceResult = twoDice()
override func viewDidLoad() {
super.viewDidLoad()
diceResult.text = "Shake em up & Roll em Dice"
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func rollOneDice() {
diceResult.text = onediceResult.oneDiceResult()
}
@IBAction func rollTwoDice() {
diceResult.text = twodiceResult.twoDiceResult()
}
}
=========
import GameKit
struct oneDice {
let choices = ["One", "Two", "Three", "Four", "Five", "Six"]
func oneDiceResult() -> String {
let onediceresult = GKRandomSource.sharedRandom().nextInt(upperBound: choices.count)
return choices[onediceresult]
}
}
=========
import GameKit
struct twoDice {
let choices2 = ["Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Eleven", "Twelve"]
func twoDiceResult() -> String {
let twodiceresult = GKRandomSource.sharedRandom().nextInt(upperBound: choices2.count)
return choices2[twodiceresult]
}
}
======
The app compiles and runs but the buttons do not show a random number when clicked. Thank you!
5 Answers
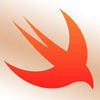
Jeff McDivitt
23,970 PointsUpload your project to Github so we can view the entire project

Luc Bernardin
3,726 PointsI'd say you forgot to create the Button IBOutlets in your viewcontroller file. Ctrl drag from your buttons into the source code below the label IBOutlet. Make sure you hook up the buttons to the IBAction functions.
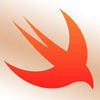
Jeff McDivitt
23,970 PointsDo you have a GitHub account?

Manik Mehta
Courses Plus Student 2,729 PointsI just created one and uploaded a .zip file into a new repository, Whatever that means. I was just trying to figure it out. My username is FreshKoder
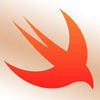
Jeff McDivitt
23,970 PointsDo you have link to it?

Manik Mehta
Courses Plus Student 2,729 Points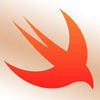
Jeff McDivitt
23,970 PointsWhat version of Xcode are you using?

Manik Mehta
Courses Plus Student 2,729 Points8.3.3
Manik Mehta
Courses Plus Student 2,729 PointsManik Mehta
Courses Plus Student 2,729 Pointshow do i do that?