Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial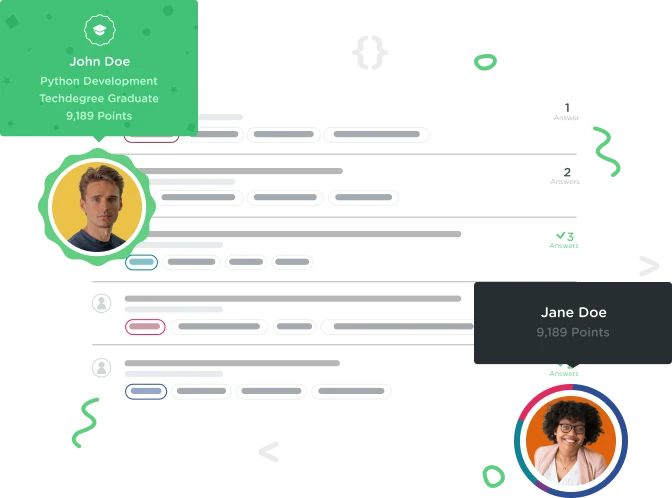
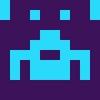
Spacey Bread
6,256 PointsI need help
please tell me what I got wrong
class GoKart {
private String color = "red";
public String getColor() {
return color;
}
public String GoKart(String color) {
}
}
2 Answers

Charlie Gallentine
12,092 PointsI'll do my best to clear it up, I am happy to offer further clarification if needed!
Part 1.
class GoKart {
private String color = "red";
public String getColor() {
return color;
}
// First task asks for the constructor
public GoKart(String color) {
}
}
The first task asks for a constructor which is empty. A constructor is formed, more or less, by giving the privacy level (public or private) and the name of the class. In the parameters is a value which was requested, in this case, a String named 'color'. This could theoretically be named anything as long as types agree, but is named 'color' for clarity.
A good general form for a constructor is:
class className { // The class definition
int memberInteger;
String memberString;
public className(int parameterInteger, String parameterString) {
this.memberInteger = parameterInteger;
this.memberString = parameterString;
}
/* When the constructor is called, it will create a new instance of the class
with the member variables set to the parameters given in the constructor */
}
//To make a new GoKart ========================
className newClassName = new className(4, "class string");
Part 2.
class GoKart {
private String color="red";
public String getColor() {
return color;
}
// First task asks for the constructor
public GoKart(String color) {
this.color = color; // Second task asks to set member variable color
}
}
The second task asks for the member variable to be set to the String in the parameters ('color'). The 'this' keyword, defines the scope of the variable being changed. In this case, when the constructor is called, it passes the value in the parameters into the String member variable 'color'. The 'this' keyword allows for only changing the color of the instance of GoKart created when calling the constructor.
class GoKart {
private String color; // Third asks to remove initialization
public String getColor() {
return color;
}
// First task asks for the constructor
public GoKart(String color) {
this.color = color; // Second task asks to set member variable color
}
}
The last task asks for the initializer to be removed. This is because it is set by the constructor. As long as its type agrees with the value which is passed to it, it will be set to whatever it is passed.
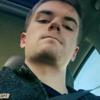
Kyle Shifflett
8,496 PointsI'm not sure what the question is asking, but looks like you need to implement the constructor maybe?
public String GoKart(String color) {
this.color = "red";
}
So, instead of declaring the variable red as an instance variable, leave it as is and allow the constructor to handle the job of assigning color to red.
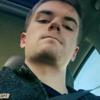
Kyle Shifflett
8,496 PointsAlso...
private String color;
leave unassigned maybe?
Spacey Bread
6,256 PointsSpacey Bread
6,256 PointsThanks a lot for the explanation I really appreciate it