Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial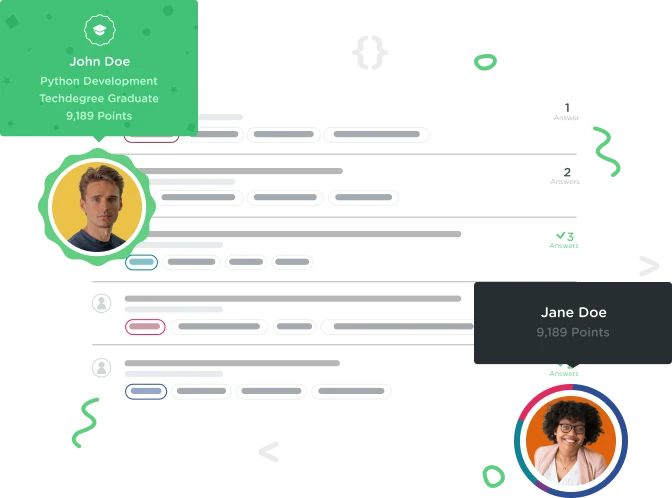

OWEN LINES
414 PointsI keep on getting an File "", line 4, in EOFError: EOF when read however I can't find the error, am I missing something?
def suggest(product_idea):
return product_idea + "inator"
try:
product_name = input("what is your idea? ")
if len(product_name) <= 3:
raise ValueError
except ValueError as err:
print("to little characters entered{}".format(err))
else:
print(suggest(product_name))
2 Answers
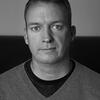
Andy Hughes
8,479 PointsOWEN LINES - Hi, I think you're overthinking what you've been asked to do. In essence all you're being asked is to do a check against product_idea
to see if it is less than 3 characters. So this should be <
and not <=
as this is 3 or less. The return statement will hold the output from the function, so this is a bit confusing as this actually will go at the end. Your code goes in between the function definition and the return statement.
To pass the challenge, you don't need to code an input so remove this code:
product_name = input("what is your idea? ")
also, the entire TRY/EXCEPT block is not needed and is incorrectly indented. The IF/ELSE is good enough, but you're missing the ELSE part, which would be the return statement. Finally, keep an eye on your indenting to make sure things are in the right place. Using an online code compiler/checker is really useful as it tells you if the indentation is incorrect.
In summary,
- Insert your code between the function name and return statement.
- An IF statement to check if
product_idea
is less than 3 characters. - If it is below 3, raise your ValueError
- An ELSE statement that uses the original return statement from the challenge.
The code below passes the challenge if you want the answer.
def suggest(product_idea):
if len(product_idea) <= 3:
raise ValueError
else:
return product_idea + "inator"
**** Update ****
It seems there might be an error with the challenge. The text says is less than 3 characters long
which should mean that the code has to be <
. But actually, the challenge also passes with <=
. Just for info.
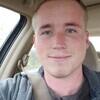
ethan wolfe
5,717 Pointsugggggghhhhh i spent hours on this and this is the only thing that works
def suggest(product_idea): if len(product_idea) < 3: raise ValueError("please enter more than 2 characters") return product_idea + "inator"
why does this code work but none of the others do? i don't know man i just bashed my head against the keyboard for hours hoping it would work. if i use else/elif/try or literally any other keywords it returns a syntax error. but if you haven't found the answer yet this will get you through lol.