Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial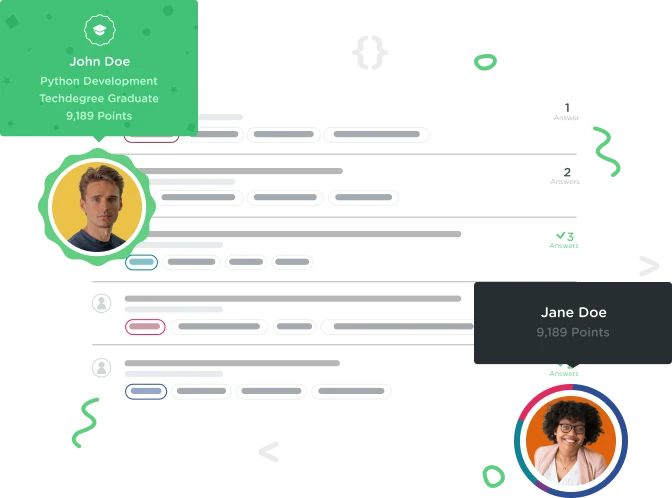
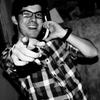
Arturo Gutierrez
11,414 PointsI have no idea how to function O_O
I am suppose to make an argument with an if condition but I'm still new to JavaScript.
function max(25,23) {
return max;
}
1 Answer

Tobias Helmrich
31,603 PointsHey there Arturo,
firstly you have to specify two parameters for the max function in the parenthesis but you're specifying values right now. You can basically name the parameters however you want but in the code below I'm using num1 and num2.
Then you have to write an if with a condition, you write the condition in the parenthesis behind the if, and then inside of the curly braces of the if you can return the greater number - if the condition is true. If not you can write an else if that checks if the other argument is greater and if that is true you can return the other number. Note that you could theoretically also write an else clause instead of an else if clause but this would also be executed if the two numbers are equal.
Here is a working example:
function max(num1, num2) {
if(num1 > num2) {
return num1;
} else if(num2 > num1) {
return num2;
}
}
I hope that helps, if you don't understand something or if you have further questions feel free to ask! :) Good luck!
Joseph Rimar
17,101 PointsJoseph Rimar
17,101 PointsThe only problem with using an else if clause here is that your function will return nothing if the numbers are equal. If you're doing it this way I would suggest adding another conditional to address equal numbers. Personally I would probably just do this to keep it simple.