Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial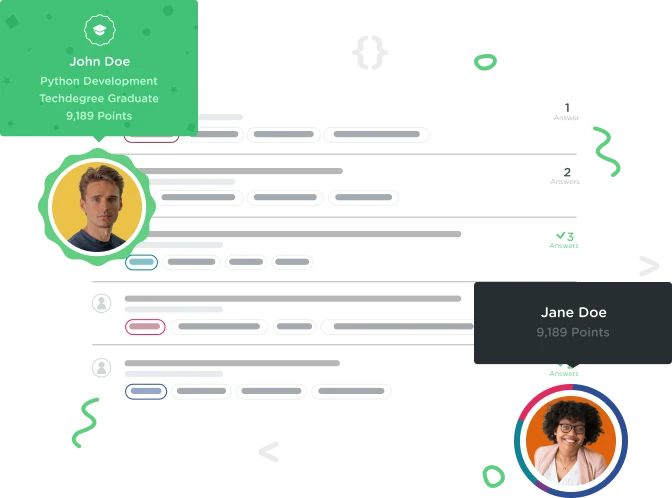

Nikolai Pütz
965 Pointsi have no idea :(
I searched on the internet and everything i understand that if you have a character you can just do character.isLetter but with a string i dont know how to do it. I saw some people did it with [a-z] stuff. I hope somebody can explain me how to do it.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
private String normalizeDiscountCode ( String discountCode){
String normalizeDiscountCode = discountCode.toUpperCase();
return normalizeDiscountCode;
}
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
discountCode = normalizeDiscountCode(discountCode);
this.discountCode = discountCode;
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer

Alexander La Bianca
15,960 PointsHi Nikolai,
What you can do is to loop through the length of the discountCode using a while loop. Here is what this code could look like
private String normalizeDiscountCode(String discountCode) throws IllegalArgumentException{
int i = 0; //initialize counter to start at the beginning of the discountCode
while(i < discountCode.lenght()) { //while loop through the discountCode to validate each character
char nextLetter = discountCode.charAt(i);
if(Character.isLetter(nextLetter) || nextLetter == '$') {
i++ ; //if it is either a letter or $ then increase the counter to get the next letter.
}
else {
IllegalArgumentException exc = new IllegalArgumentException("Invalid discount code"); //throw the exception
throw exc;
}
}
return discountCode.toUpperCase(); //this is only reached if the exception is never thrown meaning a valid discountCode
}
You can think of a string like an array that you can loop through and then treat each index like a char. You were close by knowing about the Character.isLetter() method.