Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial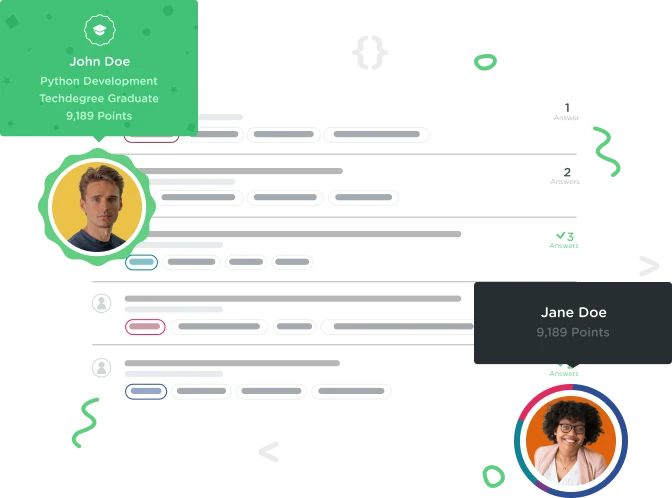

John Weland
42,478 PointsI have animation and rotation but not postilion movement.
So this will be difficult to help with I am sure but I decided to make my own game. I, having completed this section before the revamp. Came back to refresh. I have a character I can get inputs and play the animations, and rotate the player but there is no actual position movement within the world.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
public float movementSpeed;
private Vector3 movement;
private float turningSpeed = 20f;
private Rigidbody playerRigidBody;
// Use this for initialization
void Start()
{
// Gather components form the player GameObject
playerAnimator = GetComponent<Animator> ();
playerRigidBody = GetComponent<Rigidbody> ();
}
// Update is called once per frame
void Update()
{
// Gathering user input form the keyboard
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
// Use this for physics based calculations
void FixedUpdate()
{
// If the players movement vector does not equal 0 ...
if (movement != Vector3.zero) {
// ... Create a target rotation based on the movement vector ...
Quaternion targetRotation = Quaternion.LookRotation(movement, Vector3.up);
// ... and create another rotation that moves from the current rotation to the target rotation.
Quaternion newRotation = Quaternion.Lerp(playerRigidBody.rotation, targetRotation, turningSpeed * Time.deltaTime);
// ... and change the players rotation to the new incremental rotation
playerRigidBody.MoveRotation(newRotation);
// ... Then play the run animation.
playerAnimator.SetFloat("Speed", movementSpeed);
} else {
// Otherwise, don't play the run animation.
playerAnimator.SetFloat("Speed", 0f);
}
}
}
2 Answers

Oğuzhan Emre Özdoğan
3,579 PointsThis is in fact very easy to help, you used movementSpeed variable to set the speed of the frog. However, movementSpeed is NULL, you forgot to initialize it and assign a float value to it. You can do that, but there is an easier solution. Just change the movementSpeed in playerAnimator.SetFloat to 3f
// ... Then play the run animation.
playerAnimator.SetFloat("Speed", 3f);
And get rid of the movementSpeed variable completely because you will not need it anymore.
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
private float turningSpeed = 20f;
private Rigidbody playerRigidBody;
If you want to use the movementSpeed variable instead, avoid what I typed and just add this code. This will assign movementSpeed a float value of 3 and your script will work just fine:
void Start()
{
// Gather components form the player GameObject
playerAnimator = GetComponent<Animator>();
playerRigidBody = GetComponent<Rigidbody>();
movementSpeed = 3f;
}
Hope this helps!

John Weland
42,478 PointsI am not sure if its proper but it works. I have to add a Move Position to the rigid body as well.
playerRigidBody.MovePosition(transform.position + (movement * movementSpeed * Time.deltaTime));
specifically here after the rotation.
// ... and change the players rotation to the new incremental rotation
playerRigidBody.MoveRotation(newRotation);
playerRigidBody.MovePosition(transform.position + (movement * movementSpeed * Time.deltaTime));
John Weland
42,478 PointsJohn Weland
42,478 PointsHey, thank you for the response. I am actually setting the movementSpeed in the inspector, but for clarity sake I did try to set it in code instead and got the same results.