Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial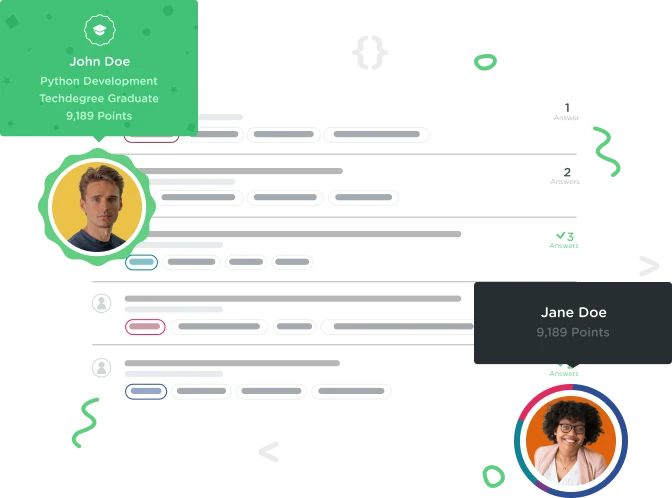

geehun seng
1,705 PointsI don't what the error in this code. Please help me to find it
In the following tasks you'll be required to select various elements on the index.html page.
In the app.js file on line 1, select all links in the nav element and assign them to navigationLinks.
let navigationLinks = document.querySelector("nav").li;
let galleryLinks;
let footerImages;
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Nick Pettit | Designer</title>
<link rel="stylesheet" href="css/normalize.css">
<link href='http://fonts.googleapis.com/css?family=Changa+One|Open+Sans:400italic,700italic,400,700,800' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<link rel="stylesheet" href="css/responsive.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<header>
<a href="index.html" id="logo">
<h1>Nick Pettit</h1>
<h2>Designer</h2>
</a>
<nav>
<ul>
<li><a href="index.html" class="selected">Portfolio</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
</ul>
</nav>
</header>
<div id="wrapper">
<section>
<ul id="gallery">
<li>
<a href="img/numbers-01.jpg">
<img src="img/numbers-01.jpg" alt="">
<p>Experimentation with color and texture.</p>
</a>
</li>
<li>
<a href="img/numbers-02.jpg">
<img src="img/numbers-02.jpg" alt="">
<p>Playing with blending modes in Photoshop.</p>
</a>
</li>
</ul>
</section>
<footer>
<a href="http://twitter.com/nickrp"><img src="img/twitter-wrap.png" alt="Twitter Logo" class="social-icon"></a>
<a href="http://facebook.com/nickpettit"><img src="img/facebook-wrap.png" alt="Facebook Logo" class="social-icon"></a>
<p>© 2016 Nick Pettit.</p>
</footer>
</div>
<script src="js/app.js"></script>
</body>
</html>
4 Answers

Robert Stewart
11,921 PointsYou're on the right track except that for the following:
// This returns a collection that contains one element, the nav element. You want the anchor tags.
// JavaScript doesn't have an .li parameter or function. JavaScript has no idea what to do with that.
let navigationLinks = document.querySelector("nav").li;
let galleryLinks;
let footerImages;
What you want to do is, get the list items from the nav element:
let links = document.querySelector('nav ul').children
This will give you a collection of 3 list items. Now, you can't do this:
let links = document.querySelector('nav ul li').children
Why not? Because it will only return the first anchor tag, not the other two. So what you have to do is then loop through each of the list items in the collection of 3 above and then add their anchor children into the navigationLinks variable. See the code below:
// We make this an array so that the .push() function will work.
let navigationLinks = [];
let galleryLinks;
let footerImages;
// We get the list items from the nav element.
let links = document.querySelector('nav ul').children
// We loop through each list item and get the anchor tag from it.
for (let i = 0; i < links.length; i++) {
navigationLinks.push(links[i].querySelector('a'));
}
I hope that helps.

geehun seng
1,705 PointsThank you so much
Chris Grazioli
31,225 Pointswhats up with the question asking for navigationLinks and the code being given as const navigationLink ??? anyone notice that screwyness?
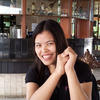
leahjoyce
6,753 Pointsgeehun seng in the first problem you're required to select all links in the nav element and assign them to navigationLinks.
My solution would be: let navigationLinks = document.querySelectorAll("nav a"); I used the querySelectorAll because I want to select all the elements inside a tag. Hope that helps you.
geehun seng
1,705 Pointsgeehun seng
1,705 Points@Robert Stewart what does .children mean?
Robert Stewart
11,921 PointsRobert Stewart
11,921 Points.children tells javascript to get the child elements of the selected element. For example, it will get all the list items (li) within a list (ul or ol). Any item that is nested inside of an element like that is called a child element, or the children of that element.