Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial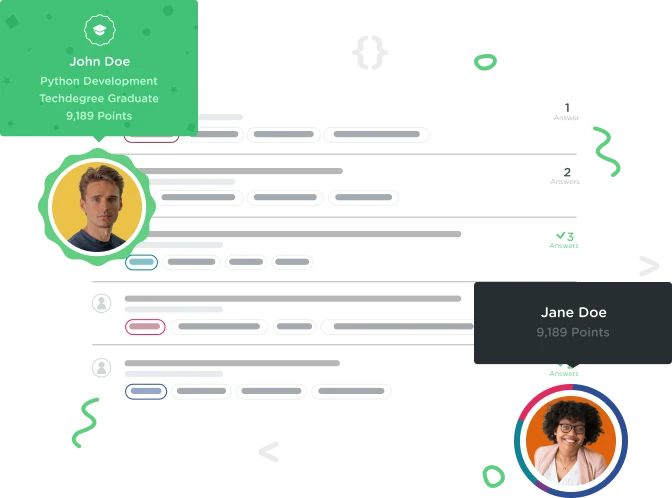

deandede
1,433 PointsI dont understand why my code is wrong
I have done this correctly and the preview doesnt show any sort of compiling errors so far...yet it says to use equalsIgnoreCase to the firstExample....i really dont understand why this is happening
Craig Dennis
Dear Sir pls help me fix this and tell me why and where i went wrong with this code challenge.
// I have imported a java.io.Console for you, it is named console.
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if (firstExample.equalsIgnoreCase(thirdExample)){
console.printf("First and Third are the same ignoring case");
}
3 Answers

andren
28,558 PointsYour code does fit the criteria for task 2, but you should take a look at the message that is present on all of these challenges right above the workspace:
In each task of this code challenge, the code you write should be added to the code from the previous task.
When doing multi-part challenges you usually have to keep the code you wrote in all of the parts to pass to the next part. In your solution you no longer have the code you wrote for the first task, that is what it causing an issue.
If you leave the code from the first task in like this:
// I have imported a java.io.Console for you, it is named console.
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if (firstExample.equals(secondExample)) {
console.printf("first is equal to second");
}
if (firstExample.equalsIgnoreCase(thirdExample)) {
console.printf("first and third are the same ignoring case");
}
Then your code will pass. It's worth mentioning though that when you remove code from a previus task the challenge checker is supposed to warn you about that in its error message. The error message you get on this task for not including the code from task 1 is quite misleading, which is likely a bug.

deandede
1,433 PointsThis code is how you show me if (firstExample.equals(secondExample)) { console.printf("first is equal to second"); }
But what actually I did was *******************
if (firstExample == secondExample) { console.printf("first is equal to second"); }
Is this wrong...I never got any sort of Error though...but ur way is also another way...pls can you tell me the difference of using both ways

andren
28,558 PointsIn this specific example either way works. But in general you should always use the equals
method when comparing strings. Using ==
will not always work with strings for reasons that are somewhat complicated to explain.
This is a bit complex for where in Java you currently are so it's possible and completely fine if you don't understand the following but I'll say it anyway just so you have some info on this. When you create a variable you are actually creating a spot in memory where the contents of that variable is stored. When you use ==
it checks to see if the two variables point to the same location in memory (meaning they are the same object), it does not check if it actually contains the same contents. As a consequence you can have two strings with the same content that will not be seen as equal by an ==
operation due to them pointing to different places in memory.
This is sometimes not an issue since Java tries to be smart about how it allocates memory and will usually point string variables to the same point in memory if it sees that they contain the same contents, but you can run into situations where that isn't the case. This is a bit simplified but it should give you the right idea.
This problem is avoided by using the equals
method since that does check the contents and not the memory location the strings points to.
It is also worth mentioning that the code checker for these challenges is far from perfect, I have seen it accept faulty code numerous times. Sometimes it will even accept faulty code for one task and then choke on that very same code when it tries to verify another task in the challenge. The point being that you should not have absolute trust that if your code passes the code checker then it definitively does exactly what it is supposed to. The code checker is usually correct, but it's certainly not flawless.

deandede
1,433 PointsThanks bro...thanks alot and 1 more thing...if I compare using .equals then how to check not equals...like i mean is that == this is equals != not equals .equals = equal then how to do the opposite of the same. using the .equals. Sorry that iam being such a pain...the main thing is I dont want to rush in Java...I do my very best to understand the very basic fundamentals bro.

andren
28,558 PointsYou can reverse the meaning of a condition using the !
operator, like this:
String example1 = "Hello";
String example2 = "World";
if (!example1.equals(example2)) {
console.printf("This will be printed out");
}
In the above example it takes the result of example1.equals(example2)
which is false and reverses it so that it actually returns true. The !
operator does not just work with equals
comparisons, you can use it to reverse any expression that returns a Boolean value.
deandede
1,433 Pointsdeandede
1,433 PointsThanks alot bro @andren, This worked out...and yeah like you mentioned it should be done otherwise it is misleading.