Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial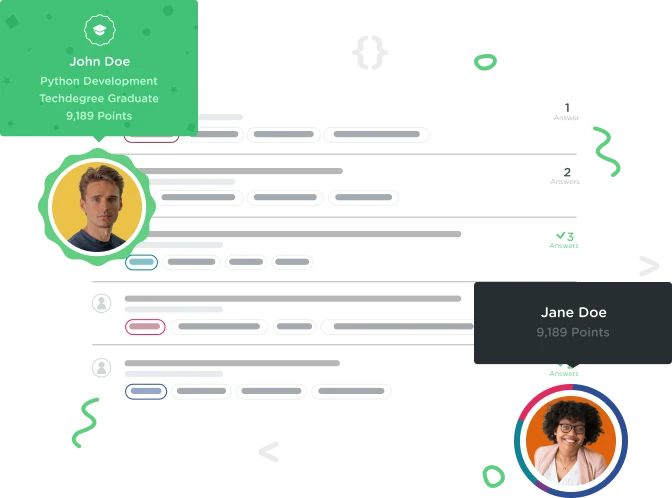

Ian Warner
1,440 PointsI don't understand part 3 of this question at all. There does not seem to be a good answer anywhere on the forum.
package com.example;
import java.util.Date;
public class BlogPost { private String mAuthor; private String mTitle; private String mBody; private String mCategory; private Date mCreationDate;
public BlogPost (String author, String title, String body, String category, Date creationDate) { mAuthor = author; mTitle = title; mBody = body; mCategory = category; mCreationDate = creationDate; } }
public String getAuthor() { return mAuthor; }
public String getTitle() { return mTitle; }
public String getBody() { return mBody; }
public String getCategory() { return mCategory; }
public Date getCreationDate() { return mCreationDate; }
}
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost (String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
2 Answers
Rebecca Rich
Courses Plus Student 8,592 PointsSo you're code looks right, minimum needed to complete the challenge was:
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
this.mAuthor = author;
this.mTitle = title;
this.mBody = body;
this.mCategory = category;
this.mCreationDate = creationDate;
}
public String getBody() {
return mBody;
}
}
Are you confused as to why you need a getter method? If so, the member variables for the BlogPost class are all private. That means that if you create a BlogPost object, you will not be able to access those variables without some public way of getting to them. That is why you need the getter methods. By creating a public method (for both getters and setters), we can control what private BlogPost variables can be get or set and how we will allow them to be get or set.
Do you have any additional questions about this challenge or Java objects in general?

Ian Warner
1,440 PointsThank you so much