Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial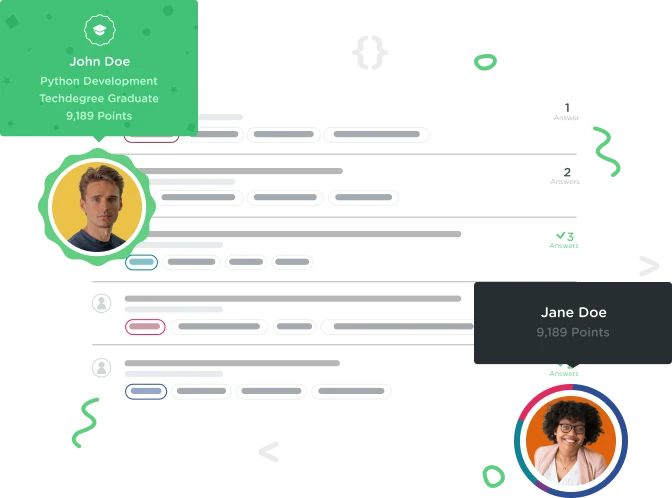

Shirit Dori
791 PointsI don't understand my problem here
What am i doing wrong?
class Example {
public static void main(String[] args) {
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try{kart.drive(100);
System.out.println("this will not happen");
}catch (IllegalArgumentException iae){
System.out.println("Whoaa there");
System.out.println("the error was ");
}
}
}
class GoKart {
public static final int MAX_BARS = 8;
private int barCount;
private String color;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
// public void drive() {
// drive(1);
//}
public void drive(int laps) {
if (laps > barCount) {
throw new IllegalArgumentException("Not enough battery remains");
}
lapsDriven += laps;
barCount -= laps;
}
}
4 Answers
Geocarlos Alves
13,969 PointsMaybe you should add the exception variable?
class Example {
public static void main(String[] args) { GoKart kart = new GoKart("purple"); if (kart.isBatteryEmpty()) { System.out.println("The battery is empty"); }
try{kart.drive(100);
System.out.println("this will not happen");
}catch (IllegalArgumentException iae){
System.out.println("Whoaa there");
System.out.println("the error was " + iae);
}
} }

Shirit Dori
791 PointsThank you Geocarlos Alves but it is still not working....

Anders Björkland
7,481 PointsOK, so this one is a bit funky, but listen. There are two things you need to do. The first one: change kart.drive(100) to kart.drive(42). That was the exercise's initial state, apparently very fond of the number 42 for some reason. The second thing: on System.out.println("the error was "); add iae.getMessage().
Good luck, Shirit!

Shirit Dori
791 PointsThank you Anders. Finally the problem was the println.It should be printf. Thanks a lot.