Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial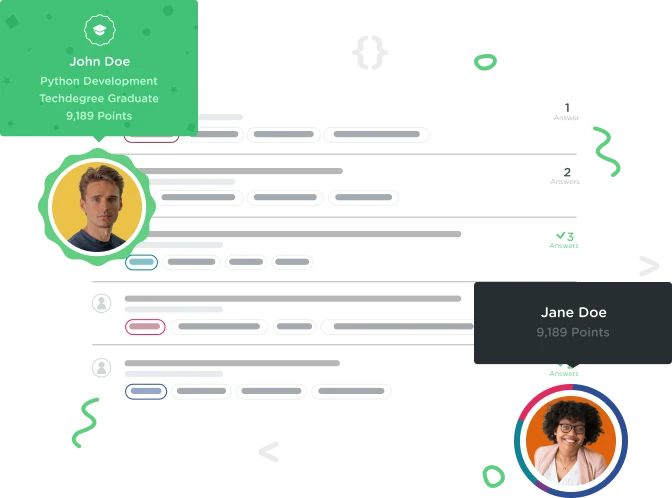

Altug Sahan
5,695 PointsI don't see why this doesn't work
The error I get is that the conditionals don't return the correct year students are in
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if(this.credits > 90) {
return 'Senior';
}
else if(60 < this.credits < 90){
return 'Junior';
}
else if(30 < this.credits < 60){
return 'Sophomore';
}
else {
return 'Freshman';
}
}
}
const student = new Student(3.9);
2 Answers

jb30
44,807 PointsTo compare three values, try changing 60 < this.credits < 90
to 60 < this.credits && this.credits < 90
. Since the previous if
statement checks if 90 < this.credits
, this else if
statement simplifies to 60 < this.credits && this.credits != 90
, since if 90 < this.credits
, it would not get to this branch. If someone with 90
credits should be a Junior
, the else if
statement could be changed to 60 < this.credits
.

Caleb Kemp
13,130 PointsThis answer was solved using the wrong parameters and has some incorrect information in it, be advised. This post has remained in its original state since there are comments below it that reference it.
So, I took a look at this for a minute and got it to work.
The first problem was that there was a syntax error with how you created the Student object, it needs both a GPA and the number of credits taken. In the example, only a GPA was given.
change
const student = new Student(3.9);
to
const student = new Student(3.9, credits);
where credits is a whole number
second part While jb30 had some great input and is correct (your code is failing to take into account edge cases), unfortunately jb30's solution isn't quite right.
To fix this with the minimal amount of change, simply change
get level() {
if(this.credits > 90) {
return 'Senior';
}
else if(60 < this.credits < 90){
return 'Junior';
}
else if(30 < this.credits < 60){
return 'Sophomore';
}
else {
return 'Freshman';
}
to
get level() {
if(this.credits >= 90) {
return 'Senior';
}
else if(60 <= this.credits < 90){
return 'Junior';
}
else if(30 <= this.credits < 60){
return 'Sophomore';
}
else {
return 'Freshman';
}
This works, however, this is not the best solution, as it has more conditions than necessary and looks confusing.
If you wanted to write a condition like else if(60 <= this.credits < 90)
it is easier to understand (and the same number of conditions) if you wrote else if(this.credits >= 60 && this.credits < 90)
. However, I think the clearest way to write this problem would look as follows.
get level() {
if(this.credits >= 90) {
return 'Senior';
}
else if(this.credits >= 60){
return 'Junior';
}
else if(this.credits >= 30){
return 'Sophomore';
}
else {
return 'Freshman';
}
This works because the if statements run in order from top to bottom. The lower else if's will only run IF, they didn't match a condition that has already run. Hope that helps, let me know if it still gives you any more difficulty.

jb30
44,807 PointsWhen I run the code const student = new Student(3.9);
, I get a student with a GPA of 3.9
and credits of undefined
rather than a syntax error.
60 <= this.credits < 90
is not the same as 60 <= this.credits && this.credits < 90
. 60 <= this.credits
is evaluated to either true
or false
, and then that boolean is converted to a number (0
if it is false
and 1
if it is true
) to compare it to 90
. Since 0
and 1
are both less than 90
, 60 <= this.credits < 90
will be true
.
For this challenge, if the student has 90
credits, the student should be a Junior
rather than a Senior
. If the student should be a Senior
with 90
credits, your final code block would work.

Caleb Kemp
13,130 Pointsjb30, I'm glad you brought that to my attention. After reviewing the code again, you're are quite right, no error is thrown by creating the object with only one value, it will give 3.9 and undefined as you mentioned. Looking at his code, I realize I assumed too much and thought the levels were supposed to be 90+,60+,30+, and under 30. However, after looking at the challenge, you are quite right, that's wrong. All the values are supposed to be offset by one like 91+,61+, etc.
Here's what it should have looked like.
get level() {
if(this.credits > 90) {
return 'Senior';
}
else if(this.credits > 60){
return 'Junior';
}
else if(this.credits > 30){
return 'Sophomore';
}
else {
return 'Freshman';
}
}
reviewing "60 <= this.credits < 90, I thought it was just an unusual way of writing it, (I didn't check if it worked, and thought he just had an edge case problem). However, you are correct, after checking, it is not the same and evaluates exactly as you described.
I greatly appreciate your input. Thanks for taking the time to comment on his post and mine.