Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial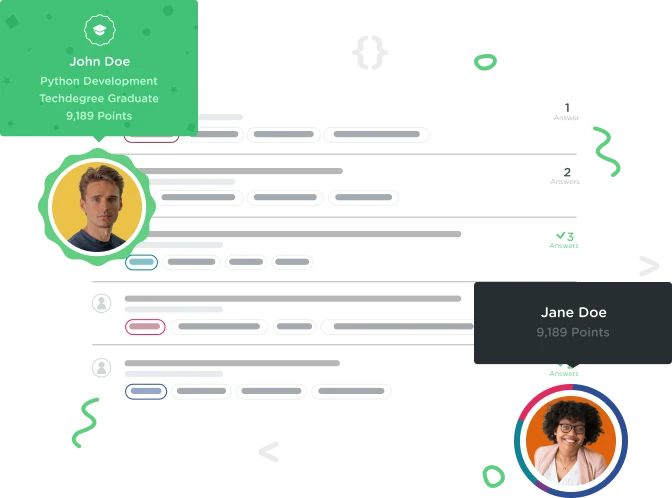
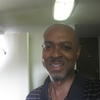
trell story
1,520 PointsI don't see the problem here
Not sure what's the problem here
import java.util.*;
public class Messy {
public static void main(String[] args) {
System.out.println("one");
System.out.println("two");
System.out.println("tree");
System.out.println("four");
System.out.println("five");
/*Please comment out this line and
this line as well with a hotkey that does multi - line commenting*/
List<String> numberWords = Arrays.asList("six", "seven", "eight", "nine");
for (String numberWord : numberWords) {
System.out.println(numberWord);
}
}
}
one
two
tree
four
five
six
seven
eight
nine
6 Answers
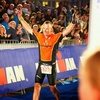
Steve Hunter
57,712 PointsOK - try changing your imports from the generic * to:
import java.util.Arrays;
import java.util.List;
Steve.
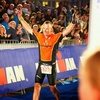
Steve Hunter
57,712 PointsAnd maybe include the whole output:
"C:\Program Files\Java\jdk1.8.0_25\bin\java" -Didea.launcher.port=7532 "-Didea.launcher.bin.path=C:\Program Files (x86)\JetBrains\IntelliJ IDEA Community Edition 14.1.5\bin" -Dfile.encoding=windows-1252 -classpath "C:\Program Files\Java\jdk1.8.0_25\jre\lib\charsets.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\deploy.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\javaws.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\jce.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\jfr.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\jfxswt.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\jsse.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\management-agent.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\plugin.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\resources.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\rt.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\access-bridge-64.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\cldrdata.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\dnsns.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\jaccess.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\jfxrt.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\localedata.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\nashorn.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\sunec.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\sunjce_provider.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\sunmscapi.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\sunpkcs11.jar;C:\Program Files\Java\jdk1.8.0_25\jre\lib\ext\zipfs.jar;C:\Users\Steve\treehouse\Messy\out\production\Messy;C:\Program Files (x86)\JetBrains\IntelliJ IDEA Community Edition 14.1.5\lib\idea_rt.jar" com.intellij.rt.execution.application.AppMain Messy
one
two
three
four
five
six
seven
eight
nine
Process finished with exit code 0
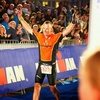
Steve Hunter
57,712 PointsFor completeness, here's my code that passes the challenge:
import java.util.Arrays;
import java.util.List;
public class Messy {
public static void main(String[] args) {
System.out.println("one");
System.out.println("two");
System.out.println("three");
System.out.println("four");
System.out.println("five");
/** Please comment out this line and
this line as well with a hotkey that does multi-line commenting **/
List<String> numberWords = Arrays.asList("six", "seven", "eight", "nine");
for (String numberWord : numberWords) {
// Use the sout shortcut to write out numberWord;
System.out.println(numberWord);
}
}
}
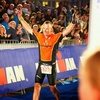
Steve Hunter
57,712 PointsHi Trell,
It might be that you're outputting "tree" not "three", perhaps?
The test is expecting a certain output which your code might not quite match. Try amending your line:
System.out.println("tree"); // small typo
I hope that helps,
Steve.
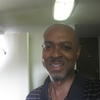
trell story
1,520 PointsThat was a good find. But It's still not working, even when corrected. Thanks again....
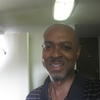
trell story
1,520 PointsI will work more with it later. But yea, I done all that you suggested already... Thanks again

Craig Dennis
Treehouse TeacherWhat error are you getting?
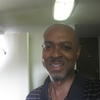
trell story
1,520 PointsBummer! Are you sure you ran the reformat code action? This seems suspect: import java.util.*
import java.util.*;
public class Messy { public static void main(String[] args) { System.out.println("one"); System.out.println("two"); System.out.println("three"); System.out.println("four"); System.out.println("five"); /Please comment out this line and this line as well with a hotkey that does multi - line commenting/ List<String> numberWords = Arrays.asList("six", "seven", "eight", "nine"); for (String numberWord : numberWords) { // Use the sout shortcut to write out numberWord; System.out.println(numberWord); } } }
I used the reformat code but I am still getting this "Bummer!" Error.. Its working on my machine fine!
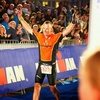
Steve Hunter
57,712 PointsHi Trell,
If you look at one of my answers above, it deals with changing the imports away from the asterisk into two specific lines of code.
Can you amend that to see if it passes the challenge, please? That's what your error is pointing at.
Steve.
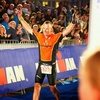
Steve Hunter
57,712 PointsCopied:
OK - try changing your imports from the generic * to:
import java.util.Arrays;
import java.util.List;
Steve.
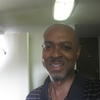
trell story
1,520 PointsThat was genius! It was the asterisk. Thanks....
Sorry about yesterday, been kinda busy..... Thanks again
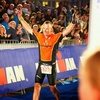
Steve Hunter
57,712 PointsCool. Glad you got it fixed.
Steve.
Eric Stermer
9,499 PointsEric Stermer
9,499 Pointsmaybe they want you to add the first five number strings to the array called numberWords. then delete the println's above it since the for loop is able to reduce the repetitive natture of all those println's.
List<String> numberWords = Arrays.asList("one", "tow", "three", "four", "five", "six", "seven", "eight", "nine"); for (String numberWord : numberWords) { System.out.println(numberWord); }
The other thing I notices was they had a comment in the original code to use the sout shortcut to output the numberWord instead of the System.out.println()