Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial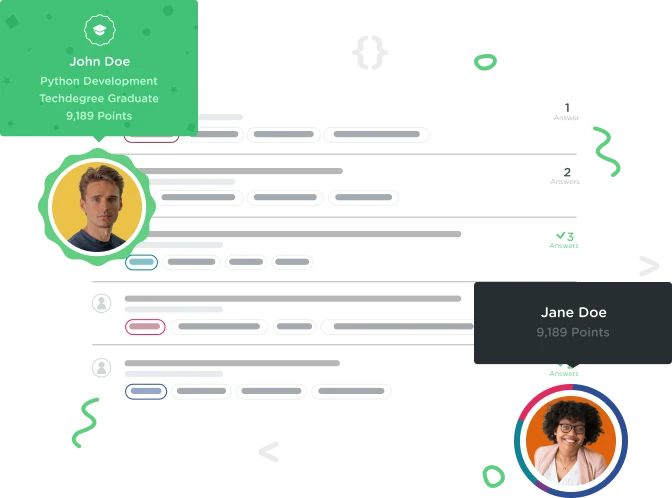

Natalia Henderson
5,190 PointsI don't know why it won't print the results
Ok, so I'm trying to build a program that can solve this problem: A researcher wants to assign 20 people to work on four projects with five people per project. The researcher asks each person to rate the projects by their level of interest, in descending order, so that the first number is their top choice and the last number the one they find least interesting. Your job is to write a program to help the researcher make up the groups fairly and based on their project preference. To do this I make up 20 random names along with a randomly generated list that represents the project preference for each person. Here is what I have so far, however, when I run it, it just outputs the print statements I have in the tester:
import java.util.ArrayList;
public class GroupPerson
{
private String myName;
private int[] myNums;
public GroupPerson( String name, int[] nums) {
this.myName = name;
this.myNums = nums;
}
public double totalScore(int []nums){
double sum = 0;
for(int i = 0; i < nums.length; ++i){
sum += (0.80 - (0.2 * i)) * Double.valueOf(nums[i]);
}
//returning summation of two values.
return sum;
}
public int[] getNum() {
return this.myNums;
}
public String getName() {
return this.myName;
}
public static void compareScore(ArrayList<GroupPerson> y, ArrayList<ArrayList<String>> x){
// put your code here
double max = 0;
double min = 0;
double interval = 0;
for(int i= 0; i < y.size(); i++){
max = y.get(0).totalScore(y.get(i).getNum());
min = y.get(0).totalScore(y.get(i).getNum());
if(y.get(i).totalScore(y.get(i).getNum()) > max){
max = y.get(i).totalScore(y.get(i).getNum());
}
if(y.get(i).totalScore(y.get(i).getNum()) < min){
min = y.get(i).totalScore(y.get(i).getNum());
}
}
interval = (max-min)/x.size();
for(int i = 0; i < x.size(); i++){
if((interval*(i) < y.get(i).totalScore(y.get(i).getNum())) && (y.get(i).totalScore(y.get(i).getNum()) < interval*(i+1))){
x.get(i).add(i, y.get(i).getName());
}
}
}
}
import java.util.ArrayList;
public class GroupPersonTester
{
public static void main( String args[] )
{
ArrayList<GroupPerson> allPeople = new ArrayList<GroupPerson>();
allPeople.add(new GroupPerson("oigs", new int[]{1, 4, 2, 3}));
allPeople.add(new GroupPerson("hgsd", new int[]{3, 2, 4, 1}));
allPeople.add(new GroupPerson("jgiu", new int[]{4, 2, 3, 1}));
allPeople.add(new GroupPerson("qwif", new int[]{3, 1, 2, 4}));
allPeople.add(new GroupPerson("kjdk", new int[]{2, 1, 4, 3}));
allPeople.add(new GroupPerson("poih", new int[]{4, 1, 3, 4}));
allPeople.add(new GroupPerson("lidu", new int[]{1, 2, 3, 4}));
allPeople.add(new GroupPerson("ibhg", new int[]{4, 3, 2, 1}));
allPeople.add(new GroupPerson("pqha", new int[]{3, 1, 4, 2}));
allPeople.add(new GroupPerson("zhyo", new int[]{2, 1, 4, 3}));
allPeople.add(new GroupPerson("mphj", new int[]{1, 3, 4, 2}));
allPeople.add(new GroupPerson("nyuz", new int[]{1, 3, 2, 4}));
allPeople.add(new GroupPerson("tnkj", new int[]{3, 4, 2, 1}));
allPeople.add(new GroupPerson("pquc", new int[]{1, 4, 2, 3}));
allPeople.add(new GroupPerson("aptr", new int[]{3, 2, 4, 1}));
allPeople.add(new GroupPerson("npqw", new int[]{4, 1, 3, 2}));
allPeople.add(new GroupPerson("dpqa", new int[]{2, 1, 3, 4}));
allPeople.add(new GroupPerson("gefk", new int[]{1, 2, 3, 4}));
allPeople.add(new GroupPerson("ynfd", new int[]{3, 4, 1, 2}));
allPeople.add(new GroupPerson("zomt", new int[]{4, 2, 3, 1}));
ArrayList<ArrayList<String>> teams = new ArrayList<ArrayList<String>>(5);
ArrayList<String> team1 = new ArrayList<String>();
teams.add(team1);
ArrayList<String> team2 = new ArrayList<String>();
teams.add(team2);
ArrayList<String> team3 = new ArrayList<String>();
teams.add(team3);
ArrayList<String> team4 = new ArrayList<String>();
teams.add(team4);
GroupPerson.compareScore(allPeople, teams);
System.out.println("Team 1: ");
for(int i = 0; i < team1.size(); i++) {
System.out.print(team1.get(i));
}
System.out.println("Team 2: ");
for(int i = 0; i < team2.size(); i++) {
System.out.print(team2.get(i));
}
System.out.println("Team 3: ");
for(int i = 0; i < team3.size(); i++) {
System.out.print(team3.get(i));
}
System.out.println("Team 4: ");
for(int i = 0; i < team4.size(); i++) {
System.out.print(team4.get(i));
}
}
}
1 Answer

KRIS NIKOLAISEN
54,970 PointsI have limited java skills and it's been awhile since I took statistics but I thought the problem interesting enough to give it a shot. Here is a snapshot and comments below:
For class GroupPerson
1) I'm not sure why totalScore
has a parameter for nums when they are already stored in this.myNums
. You could also save a lot of processing by calculating and storing the score when the person is created. Then totalScore
would just return the stored value. I did not fix this in the code I provided.
2) If you check your max and min values after the loop you will see they are the same and therefore interval is 0. Looking at your code closer you see that each time through the loop you reassign max and min to the score of the current item and then compare each to the same value. Therefore after the loop, max and min will be the score for the last item in the loop.
Although this works:
max = y.get(0).totalScore(y.get(i).getNum());
min = y.get(0).totalScore(y.get(i).getNum());
I'm not sure why you are using item 0 to calculate the score for item i. If the point of the loop is to find the min and max scores for allPeople it made more sense to me to initialize min and max outside the loop then check values and update as needed.
max = y.get(0).totalScore(y.get(0).getNum());
min = y.get(0).totalScore(y.get(0).getNum());
for(int i= 0; i < y.size(); i++){
if(y.get(i).totalScore(y.get(i).getNum()) > max){
max = y.get(i).totalScore(y.get(i).getNum());
}
if(y.get(i).totalScore(y.get(i).getNum()) < min){
min = y.get(i).totalScore(y.get(i).getNum());
}
}
3) When creating teams you have to loop through all people and check each score against the interval for each team. This can be done with two loops. It also made more sense to me that the intervals would be added to min since that would be the base. Here the outer loop is for the people and the inner loop is for the teams.
for(int i = 0; i < y.size(); i++){
for(int j = 0; j < x.size(); j++){
if((min + interval*(j) <= y.get(i).totalScore(y.get(i).getNum())) && (y.get(i).totalScore(y.get(i).getNum()) < min + interval*(j+1))){
x.get(j).add(y.get(i).getName());
}
}
}
In making these changes the teams are built and will print, but there are not exactly 5 people in each group. You will also have to deal with duplicates. For this it may be better to sort people by score and then add them start to finish in groups of five.