Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial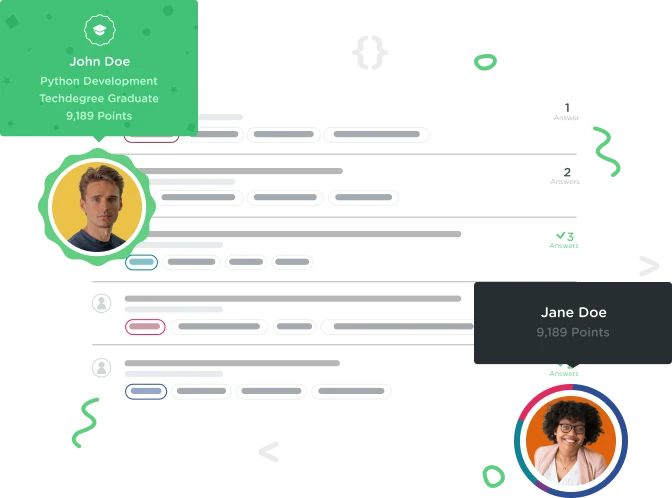

Jacinto Jacinto
Python Web Development Techdegree Student 7,869 PointsI don't know what I'm doing wrong. I get the desired output but the quiz doesn't agree for some reason.
On my side, I am able to create and instance of the Letter class with my method. I even tested all the previously defined methods out to see if it works.
bleep = Letter.from_string("dash-dot-dash-dot")
print(bleep)
Console: dash-dot-dash-dot
for i in bleep.pattern:
print(i)
Console:
-
.
-
.
empty_list = []
empty_list.extend(bleep)
print(empty_list)
Console: ['-', '.', '-', '.']
I have replicated the same tests on a variable that you would create normally without using the "from_string" method I made: bloop = Letter(pattern=["-", ".", "-", "."]) and it would output the exact same results into the console. I'm pretty sure that's what the method was aiming to do except taking in a string version instead, unless I'm completely wrong.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls, my_string):
correct_list = []
dash_dots = my_string.split("-")
for i in dash_dots:
if i == "dash":
correct_list.append("-")
elif i == "dot":
correct_list.append(".")
correct_pattern = Letter(pattern=correct_list)
return cls(correct_pattern)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
[MOD: added ```text formatting -cf]

Jacinto Jacinto
Python Web Development Techdegree Student 7,869 Pointsit did it again with "Output:". I think people will get my point though.
1 Answer
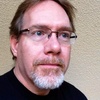
Chris Freeman
Treehouse Moderator 68,460 PointsYou are very close.
- correct the append for "dash" to be an underscore (_) instead of a hyphen (-)
- return the Letter object
correct_pattern
instead of callingcls()
Post back if you need more help. Good luck!!!

Jacinto Jacinto
Python Web Development Techdegree Student 7,869 PointsThanks for the help! What is the reason for not calling cls when returning the class and its pattern? During the video right before this quiz we had to call it for the bookcase example.
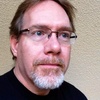
Chris Freeman
Treehouse Moderator 68,460 PointsThe parameter cls
represents the current class where this method resides. So using cls()
will call the constructor to create a new instance of that class. However, the new class was already explicitly created using Letter()
.
Using cls()
on the Letter
object in correct_pattern
will raise an error since correct_pattern
as an argument is its string representation that is just the pattern and would be missing the keyword "pattern".
Additionally, the string pattern would be back converted to "dash"/"dots" so would be of the wrong format.
An alternative that works is to use:
return cls(pattern=correct_list)
# same result as
return correct_pattern
Jacinto Jacinto
Python Web Development Techdegree Student 7,869 PointsJacinto Jacinto
Python Web Development Techdegree Student 7,869 PointsHmm that's weird, when I typed in a new line and started it with "Console:" it bugged out, enlarged itself, and deleted most of my test evidence I tried to post. That might be another bug TreeHouse needs to fix.
In any case, I will retype it to give more context. I'll just say output in place of console just in case it bugs out again.
bleep = Letter.from_string("dash-dot-dash-dot") print(bleep) Output: dash-dot-dash-dot
for i in bleep: print(i)
Output:
.
.
empty_list = [] empty_list.extend(bleep) print(empty_list) Output: ['-', '.', '-', '.']
I'll just repeat what I said on my post for the sake of being clear:
I have replicated the same tests on a variable that you would create normally without using the "from_string" method I made: bloop = Letter(pattern=["-", ".", "-", "."]) and it would output the exact same results into the console. I'm pretty sure that's what the method was aiming to do except taking in a string version instead, unless I'm completely wrong.