Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial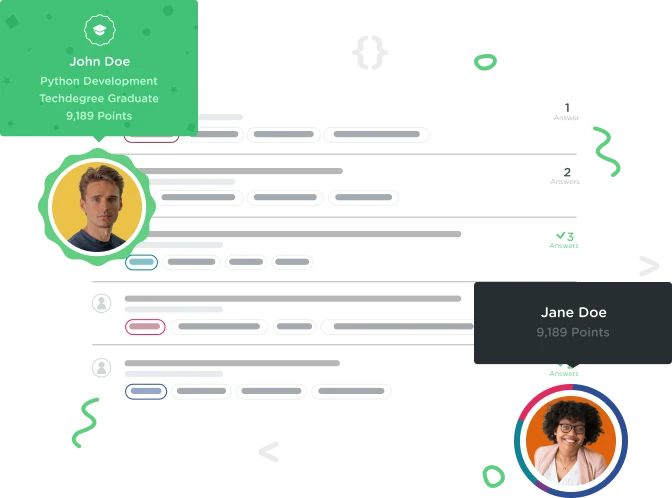
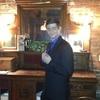
Carl Smith
8,185 PointsI don't know how to increment one integer to make it add to another.
Not sure what I need to do next?
bool levelFinished;
int rubies = 0;
int rubyReward = 7;
int rubyMax = 50;
while(rubies <= rubyMax) {
rubyReward++
}
if (rubies >= rubyMax)
levelFinished = TRUE;
1 Answer
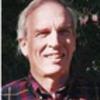
jcorum
71,830 PointsYou can do this:
rubies = rubies + rubyReward;
or this:
rubies += rubyReward;
They are equivalent.
The key thing is that you are adding rubyReward to rubies, and then assigning the result of that addition back to rubies. So if rubies is 7 and rubyReward is 7, then rubies += rubyReward would be 14.
bool levelFinished;
int rubies = 0;
int rubyReward = 7;
int rubyMax = 50;
while(rubies < rubyMax) {
rubies += rubyReward;
}
levelFinished = TRUE;
jcorum
71,830 Pointsjcorum
71,830 PointsP.S., note that in the while loop you were supposed to loop while rubies is less than rubyMax, not while rubies is less than or equal to rubyMax.
Also, your rubyReward++ code is shorthand for rubyReward = rubyReward + 1 and for rubyReward += 1