Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial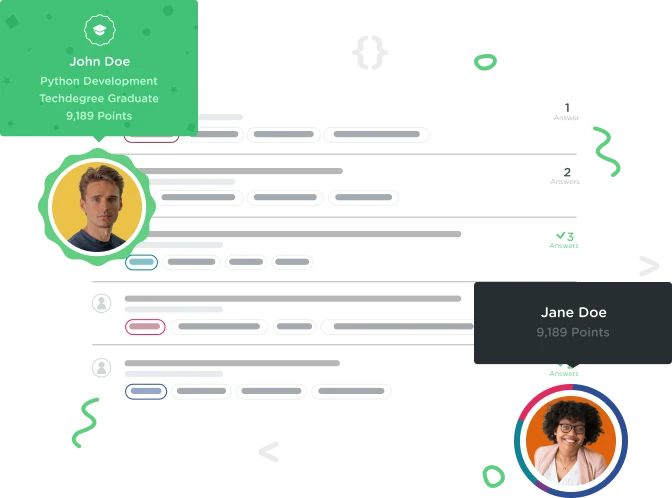

Zac Duvall
3,662 PointsI don't even know where to begin. Please help.
heres what i've written:
init(red: 86.0, green: 191.0, blue: 131.0, alpha: 1.00) {
let red = "Red"
let green = "Green"
let blue = "Blue"
let alpha = "Alpha"
}
and I get an error saying that I should have a ',' after 'alpha:'. I am hopelessly lost, there is a huge jump form the lectures to the challenge.
1 Answer

lvwdhydynw
6,443 PointsHi Zac!
Let's go step by step. At the beginning, you are given this code:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
}
First thing you have to do is this: "Your task is to write a custom initializer method for the object. Using the initializer assign values to the first four properties"
So, you have to write initializer method which should assign values to constants red, green, blue and alpha via given parameters. Imagine you were given simple struct called Person with 2 properties (name, age) and you should do the same here. Look at this:
init(name: String, age: Int) //if I want to have instances with different values in
//properties, I have to write these properties as parameters
//Here I have declared a parameter name of type String and a parameter age of type Int
{
self.name = name //I want to assign value of parameter name to the variable/constant name
//This is how you do it. We use self to help Xcode realize which
//side the variable/constant declared in this structure is
//This way we know that the name on the left is the var/let
self.age = age //Same here
}
The other task is: "sing the values assigned to those properties create a value for the description property that is a string representation of the color object".
Again, we will do the same with my made up structure Person. I want the description look like this (if the name is Marcus and the age is 23): "Hi, I am Marcus and I am 23". This is how you'd do it:
description = "Hi, I am \(name) and I am \(age)"
}
That's nothing but String Interpolation. You may find more about it here: https://developer.apple.com/library/content/documentation/Swift/Conceptual/Swift_Programming_Language/StringsAndCharacters.html
If there is something you don't understand, just ask me and I will explain it to you :)
Zac Duvall
3,662 PointsZac Duvall
3,662 PointsThis was incredibly helpful!! thank you