Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial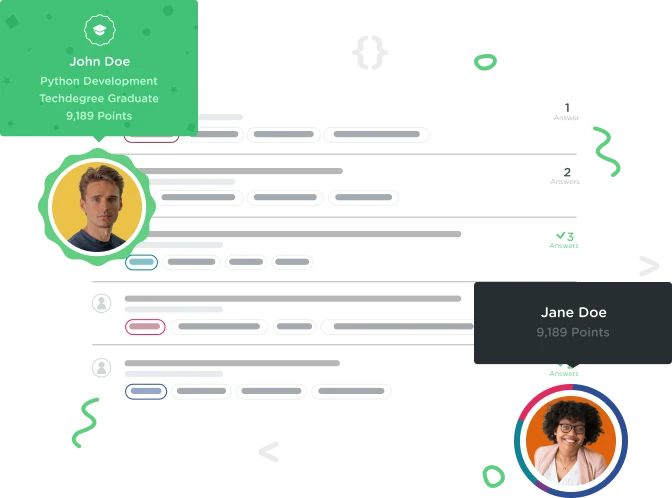

ilanaguttman
2,700 Points"I do not see the correct default output" - switch statement exercise
Why am I getting error message "I do not see the correct default output" for the switch statement exercise?
<?php
//Available roles: admin, editor, author, subscriber
if (!isset($role)) {
$role = 'subscriber';
}
//change to switch statement
$role = "admin";
switch ($role){
case "subscriber":
echo 'You are a subscriber and do not have access to this page.';
break;
case "author":
echo 'You are an author and do not have acccess to this page.';
break;
case "editor":
echo ' You are an editor and do not have access to this page.';
break;
case "admin":
echo 'You are an administrator. Welcome.';
break;
default:
echo "You do not have access to this page. Please contact your administrator.";
}
if ($role = "admin"){
echo 'As an admin, you can add, edit, or delete any post.';
}
?>
4 Answers
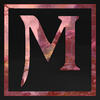
Matthew Lang
13,483 Points$role is equal to "subscriber", so the switch statement catches that and echos echo 'You are a subscriber and do not have access to this page.';. You don't want the switch statement to catch anything, so it will run 'default'.
if (!isset($role)) {
$role = 'AnythingThatIsntListedInTheSwitchStatement';
}

ilanaguttman
2,700 PointsI can't change
if (!isset($role)) {
$role = 'subscriber';
}
at the top. I'm not supposed to edit that section, and if I do, it tells me not to edit the code above the switch statement. The instructions say to keep the same default output. "Step 1: Change the second if statement to a switch statement, keeping the current message as the default."
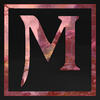
Matthew Lang
13,483 PointsIf you are on the first task, use this. It wants you to only add the 'admin' in.
<?php
//Available roles: admin, editor, author, subscriber
if (!isset($role)) {
$role = 'subscriber';
}
//change to switch statement
switch($role) {
case "admin":
echo "As an admin, you can add, edit, or delete any post.";
break;
default:
echo "You do not have access to this page. Please contact your administrator.";
}
If you're on the second task, use this. It wants you to add two additional roles, not them all.
<?php
//Available roles: admin, editor, author, subscriber
if (!isset($role)) {
$role = 'subscriber';
}
//change to switch statement
switch($role) {
case "admin":
echo "As an admin, you can add, edit, or delete any post.";
break;
case "editor":
echo "As an editor, you can add or edit any post, and delete your own posts.";
break;
case "author":
echo "As an author, you can add, edit, or delete your own post.";
break;
default:
echo "You do not have access to this page. Please contact your administrator.";
}

ilanaguttman
2,700 PointsIf you're on the second task, use this. It wants you to add two additional roles, not them all.
How did you know it only wants us to add 2 additional roles?

ilanaguttman
2,700 PointsBecause it says "Add a second check" - so logically it would make sense to have only 2 if anything.