Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial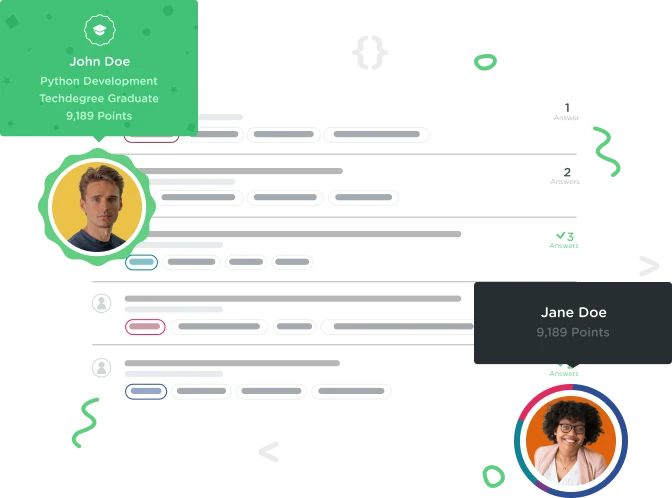

Eirin Joahna Pamela Gonzales
8,686 PointsI did not forget the playHoldMusic() in my while loop. I also used the poll() to remove the csr. Still, error.
What is wrong with my code?
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr = new CustomerSupportRep("Eirin");
mSupportReps.add(csr);
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
while ((csr = mSupportReps.poll()) == null) {
playHoldMusic();
}
//mSupportReps.poll();
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
csr.assist(customer);
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
mSupportReps.add(csr);
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
4 Answers
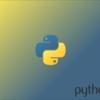
Kevin Faust
15,353 PointsHey Eirin,
I'll explain what is going on and hopefully you'll understand.
In the very top of our acceptCustomer() method, we want to check if there is a customer support rep in our queue. As you can see in your code, the very first thing you did is create a new customer support rep and then you added it to the queue. This is not what the challenge is expecting/wanting you to do.
We are not going to be the ones creating a new customer support rep or anything. our code is focusing on making sure that there is someone in the queue and then dealing with what will happen if we have someone or not in our queue. we only initialize that csr variable AFTER we make sure we have a customer rep in our queue.
As you can see, you manually added a customer rep and you assigned it to csr. csr should be assigned via poll()
This is how the first part should look like:
while (mSupportReps.poll() == null) {
playHoldMusic();
}
As you can see, we refer to our queue directly and check in our queue if there is anyone there or not. if empty, then play the music.
Now, if there is a person in our customer rep queue, then we pull them out of the queue and assign it to our csr variable as below and then we call the assist() method after that:
csr = mSupportReps.poll();
csr.assist(customer);
the rest of the code you've got it down correctly.
Happy coding and best of luck,
Kevin

Seth Kroger
56,416 PointsThere's no need to create a new rep or add it to the queue. The queue in that case would always have a rep and never play the hold music.
CustomerSupportRep csr = new CustomerSupportRep("Eirin");
mSupportReps.add(csr);
should just be the original
CustomerSupportRep csr;

Eirin Joahna Pamela Gonzales
8,686 PointsThanks Kevin! I got it! :D
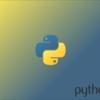
Kevin Faust
15,353 PointsGlad to have been of help :D
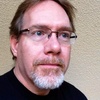
Chris Freeman
Treehouse Moderator 68,460 PointsHey Kevin F, I am working on the same code challenge and have questions about your suggested code:
while (mSupportReps.poll() == null) {
playHoldMusic();
}
and
csr = mSupportReps.poll();
csr.assist(customer);
During the while
loop test, as long as the mSupportReps
queue is empty, all is fine because mSupportReps.poll()
returns null
. However, during the first loop test when there is a rep in the queue, mSupportReps.poll()
will return a non-null value so the hold music code block is by-passed. No issue so far, until you reach the csr
assignment.
At the csr
assignment, mSupportReps.poll() is called again, but there's nothing left in the queue because it was removed during the while loop test. For example:
// in java-repl
java> import java.util.Queue;
Imported java.util.Queue
java> import java.util.ArrayDeque;
Imported java.util.ArrayDeque
java> Queue<String> mStringQueue = new ArrayDeque<String>();
java.util.Queue<java.lang.String> mStringQueue = []
java> mStringQueue.add("bob")
java.lang.Boolean res7 = true
java> mStringQueue.size()
java.lang.Integer res8 = 1
java> while (mStringQueue.poll() == null) {
| System.out.println("queue empty");
| }
java> // Queue not empty; code block skipped
java> String str1 = mStringQueue.poll();
java.lang.String str1 = null
java> // Null returned
It seems the preferred solution would be to do the assignment to csr
within the while lop test:
while ((csr = mSupportReps.poll()) == null) {
playHoldMusic();
}
This way the loop runs until the assignment to csr
is non-null and csr
retains the rep polled from the queue. Reviewing this in the repl:
// in java-repl
java> mStringQueue.add("carol")
java.lang.Boolean res10 = true
java> mStringQueue.size()
java.lang.Integer res11 = 1
java> while ((str1 = mStringQueue.poll()) == null) {
| System.out.println("queue empty");
| }
java> str1
java.lang.String str1 = "carol"
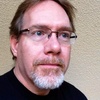
Chris Freeman
Treehouse Moderator 68,460 PointsKevin, I see in other solutions on this challenge you used .peek()
instead of .poll()
within the while
loop test. The peek
solution works, but as in your answer here, the poll
version does not pass the challenge as written.
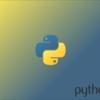
Kevin Faust
15,353 PointsHey Chris Freeman ,
Yep you're right. 2 months ago, you could use both poll() or peek() to complete the challenge. That was why I used them interchangeably in the while statement. I guess treehouse saw the flaw with this and changed it.
Good luck!!
Chris Freeman
Treehouse Moderator 68,460 PointsChris Freeman
Treehouse Moderator 68,460 PointsAs written, this does not pass the challenge. It will pass if the
.poll()
within thewhile
loop test is changed to.peek()
.