Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial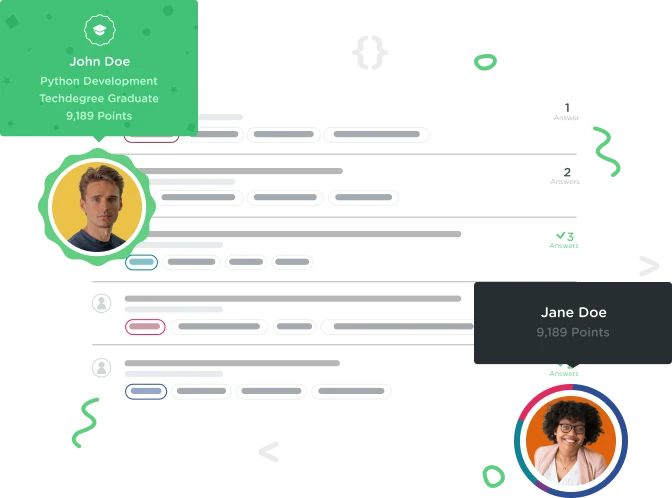

parth bhardwaj
4,353 PointsI cant find whats wrong with my code:
def reverse_evens(list4): return list4[len(list4)+1:0:-2]
def first_4(list1):
return list1[0:4]
def first_and_last_4(list2):
return(list2[0:4]+list2[len(list2)-4:len(list2)+1])
def odds(list3):
return list3[1:len(list3)+1:2]
def reverse_evens(list4):
return list4[len(list4)+1::-2]
4 Answers
Dustin James
11,364 PointsThe following will pass the challenge:
def first_4(list):
return list[0:4]
def first_and_last_4(list):
first = first_4(list)
second = list[-4:]
return first + second
def odds(list):
return list[1::2]
def reverse_evens(list):
return list[-1::-2]
The following would be test case safe:
def reverse_evens(list):
try:
if len(list) % 2 != 0:
reverse_even = list[-1::-2]
else:
reverse_even = num[-2::-2]
except ValueError:
print("Non iterable item")
else:
return reverse_even
Or even another way to solve this is to get the even indices with a slice [::2] and then return a slice in reverse order [::-1]
def reverse_evens(list):
list = list[::2]
return list[::-1]
I hope this helps.

markmneimneh
14,132 PointsHello
the code above assumes all you are always passing numeric values and it is returning the even values (not the indexes). I mis-read the question.
you case is much simpler ... this is all you need
def reverse_evens(item):
return(item[-1::-2])
this the the full 4 questions
def first_4(item):
return item[:4]
def first_and_last_4(item):
return(item[:4] + item[-4::])
def odds (item):
return(item[1::2])
def reverse_evens(item):
return(item[-1::-2])

parth bhardwaj
4,353 Pointsfor the code you have given won't work for: [1,2,3,4] it would return [4,2] it should be returning [3,1] // even indexes note: my code is working generally but it is failing for some specific test case.

parth bhardwaj
4,353 Pointsdef reverse_evens(list4): if(len(list4)%2==0): return list4[len(list4)-2::-2] else: return list4[len(list4)-1::-2] // this is the answer :)

markmneimneh
14,132 PointsHi
whether it returns even or odd, depends on you starting point. which is the first item in the :: setup; because the last part is the increment. so if you increment by 2 starting from 0, you get one list. where as if you increment by 2 stating from 1, you get another list.
The key is to understand how :: works.

markmneimneh
14,132 PointsHello
You almost there
try this ....
def reverse_evens(list4):
new_list= []
for item in list4[::-1]:
if item % 2 !=0 :
new_list.append(item)
return new_list
print(reverse_evens([1, 2, 3, 4, 5])) #<<<<<<<<< for demo only
if you are not familiar with the % operator, it returns the reminder from a div operation. You can google it.
if this answers your question, please mrk question as answered.

parth bhardwaj
4,353 PointsEven your code is showing an error: Bummer! TypeError: not all arguments converted during string formatting