Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial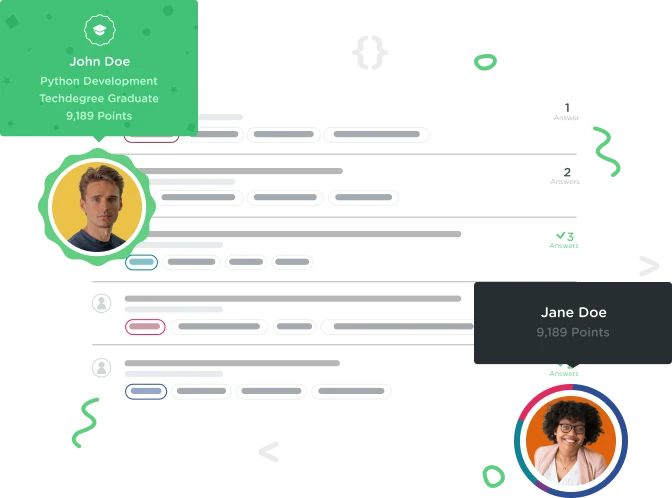
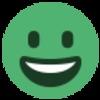
Jack Cummins
17,417 PointsI cannot figure out why my code is wrong
Alright, I need you make a new method named feedback. It should take an argument named grade. Methods take arguments just like functions do. You'll still need self in there, though.
If grade is above 50, return the result of the praise method. If it's 50 or below, return the reassurance method's result.
class Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self):
if grade > 50:
return praise()
else:
return reasurance()
5 Answers
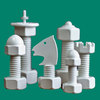
Steven Parker
241,972 PointsThe instructions say, " I need you make a new method named feedback. It should take an argument named grade."
But the function shown here doesn't take any argument (other than the implicit "self").
Also remember to use the "self." prefix when calling instance methods from within the class, and be careful about spelling!
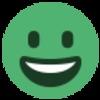
Jack Cummins
17,417 PointsI used your reccomendation and it did not work. Would you please show me the code. I will make sure to take in and absorb the code as well as I can.
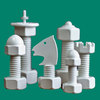
Steven Parker
241,972 PointsShow the code you have now with the argument and the "self." prefixes added and the spelling fixed and I'll take another look.
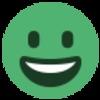
Jack Cummins
17,417 Pointsclass Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self, grade):
return "Chin up, {}. You'll get it next time!".format(self.name)
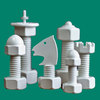
Steven Parker
241,972 PointsYou've gone way off-track here, and were closer the first time.
The instructions are to "make a new method named feedback". You won't need to make any changes to the methods that are already there.
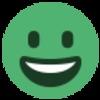
Jack Cummins
17,417 Pointsclass Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return praise()
else:
return reasurance()
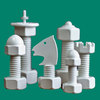
Steven Parker
241,972 PointsSo that's got the parameter added, but you still need to add the "self." prefixes to the method calls and fix the spelling.
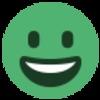
Jack Cummins
17,417 PointsI don't get it. Where am I supposed to put the self.prefixes method? I know that this is a dumb question, but I really do not get what this code challenge was asking me to do. Would you please help me?
Sorrry for my troubles, Jack
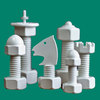
Steven Parker
241,972 PointsWhen you call an internal method, like "return praise()
", add the prefix to the method name: "return self.praise()
".
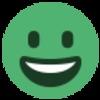
Jack Cummins
17,417 Pointsclass Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
else:
return self.reasurance()
This code doesn't pass.
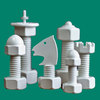
Steven Parker
241,972 PointsYou still need to fix the spelling.
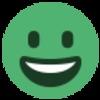
Jack Cummins
17,417 PointsThank YOU!!!!!!!!!!!!!!!!!

David Valverde
3,198 PointsThere you go!
def feedback(self, grade): if grade > 50: return self.praise() else: return self.reassurance()