Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial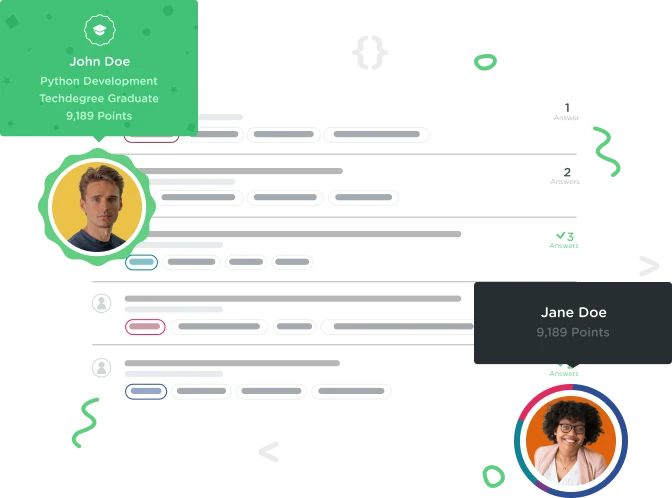

Elaine Cadman
2,335 PointsI can do this without the .forEach, but I am lost with the .forEach
for(var i=0; i<numbers.length; i++) { times5[i] = numbers[i] * 5; }
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
// times5 should be: [5,10,15,20,25,30,35,40,45,50]
// Write your code below
for(var i=0; i<numbers.length; i++) {
times5[i] = numbers[i] * 5;
}
3 Answers

James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsHi Elaine,
You could try something like:
numbers.forEach(function(number){
times5.push(number * 5);
});
forEach is an array method, so it needs to be attached to an array
numbers.forEach()
To access each number we use the function parameter. We can call this whatever we want, in this case I chose number as its the singular version of 'numbers'.
numbers.forEach(function(number)) { }
Then we multiple the number by 5 and push it to the times5 array
times5.push(number * 5);
See MDN - forEach
Hope this helps :)

Gabriel Plackey
11,064 PointsFor each is kinda like shorthand for a for loop. So you can the same thing while writing less code.

Elaine Cadman
2,335 PointsYES James Anwyl very helpful, I am truly grateful for your help!

James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsNo problem :)
Elaine Cadman
2,335 PointsElaine Cadman
2,335 Pointsomg thank you, this makes so much sense. Javascript syntax is hard to adjust to! I know what I want to do, but doing it is proving tricky!
Elaine Cadman
2,335 PointsElaine Cadman
2,335 Pointsbut how does it know "number" instead of "numbers"?
James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsJames Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsIf you look at the link I posted under 'syntax'
You can see that the first argument to the function is the current value of the array.
Each time around the loop currentValue will change. The first time around the loop, currentValue is equal to the first item in the array. Second time around the loop, currentValue is equal to the second item in the array...
We can name the current value whatever we want, writing:
or
Is just as valid. However, you want to call it something that is meaningful and descriptive.
Hope this helps :)