Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial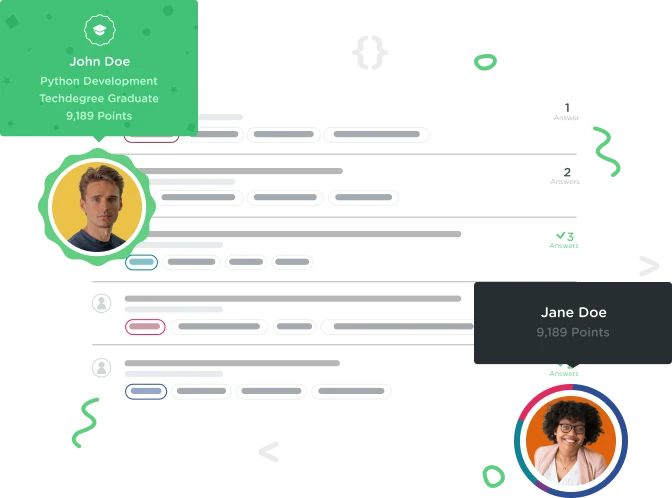

Roshan Kashif
2,976 PointsI am totally stuck
what am I meant to do in this challenge
package com.example.model;
import java.util.List;
import java.util.Set;
public class Course {
private String mTitle;
private Set<String> mTags;
public Course(String title) {
mTitle = title;
// TODO: initialize the set mTags
}
public void addTag(String tag) {
// TODO: add the tag
}
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
}
public boolean hasTag(String tag) {
// TODO: Return whether or not the tag has been added
return false;
}
public String getTitle() {
return mTitle;
}
}
4 Answers
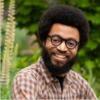
Nicolas Hampton
44,638 PointsOk, without doing the exercise for you or looking up the instructions that you left out, I still understand why this looks difficult. Break it down into smaller parts. If you come out of this course learning one thing, it should be to break things down into manageable parts. The first thing we need to fill in has the same name as the Class we're working in, Course. This is the constructor, and the constructor initializes (or sets a value to) all the values this object (and think of the word object as literal) has. Tags, or mTags, sounds a little confusing, so think of the course we're taking. This course is made up of 10 or so videos. So we could say that a course is a collection of educational videos, or in the case of this program, a collection of tags. The constructor they have written here takes the title of the course as the argument (the part in parenthesis) . They didn't give us any tags! So what we're going to do is simply make a blank list that we can put tags in later. Above the constructor there's a couple of member variables and, low and behold, there's a Set of Strings called mTags. But a set is just the interface, we need the implementation stored inside of it. So, for the Course constructor, all we need to do is store a blank implementation into the member variable mTags. That should finish the constructor. Don't worry about the title yet, that's been done for you.
Now we have a title for the course and a blank set of tags that makes up every object in the course class (the one we just defined). Next they want us to make a method to add tags to the blank set of tags we just made, or mTags. The argument they have made the method take is a String called Tag. Make the code to "add" the tag coming into the method to the set of mTags. One line of code should do it, and the method says void, so it returns nothing. Just "add" a Tag to the set of mTags.
Adding one tag at a time seems tedious, so they're asking us to make a method to add a Set of tags all at once, or addTags. This is a little trickier. Seems as though we would have to add one tag at a time to mTags, but the argument is a list of tags. How do we add them all at once. I don't even know how big the list is that's coming in! Then let's not add them all at once. Lets just add them one by one until we run out of tags. We already made a method for that anyways, we just have to LOOP through each TAG in the LIST and addTag each one. Remember to keep the code DRY, don't repeat yourself. The easiest way to do this is to use the small things we've already done in what we have to do. Hint: learn to love the for loop.
The last thing that has to be made is the hasTag method. Craig has named it hasTag to be self explanatory. We just made this course object with a whole set of tags, he just wants to know if the tag we pass into hasTag is in the course we're taking. Remember, this is in the Course class, so in code, we would use this method as someCourse.hasTag("tag"), meaning we're looking for this particular tag in the list of tags in someCourse he's teaching. This needs one line of code, but off the top of my head, I don't know how to find a single string in a set of strings. But I'm not afraid to look it up. A great way to do this is to check out the java documentation at oracle: http://docs.oracle.com/javase/7/docs/api/java/util/Set.html One of the built in methods for Sets has to be able to find a particular item in a set, right? Don't ever be afraid to refer to this. Remember, it's not cheating if the job gets done.
These are all really small tasks that look really big all together. Break them up. Break up the programs as much as you can, we can use them more ways in the future if you break up what everything does as small as possible. It makes it easier to read and easier to fix. I hope that was helpful, and if you get stuck, well ask again. Good luck! Nicolas

Andre Colares
5,437 Points public Course(String title) {
mTitle = title;
// TODO: initialize the set mTags
mTags = new HashSet<String>();
}
public void addTag(String tag) {
// TODO: add the tag
mTags.add(new String(tag));
}
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String allTags : tags){
mTags.add(new String(allTags));
}
}
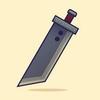
Allan Clark
10,810 PointsYou have three methods to fill in addTag, addTags, and hasTag.
1) for addTag: You receive a String called tag, and need to add it to the Set of Strings called mTags. Here is the doc for Set, try to find a method that will add a single object to the set. http://docs.oracle.com/javase/7/docs/api/java/util/Set.html
2) for addTags: You receive a List of Strings called Tags and need to add them all to the mTags Set. Easiest thing do here would be to create a loop that goes to the end of the tags List and calls addTag for each. This can be done with a for or for each loop (which ever you prefer). Here is the List documentation: http://docs.oracle.com/javase/7/docs/api/java/util/List.html
3) for hasTag You just want to check if the mTags Set contains the given tag. This can be done by a method in the Set class. http://docs.oracle.com/javase/7/docs/api/java/util/Set.html

Kyle Baker
1,884 PointsIn the documentation you posted, (http://docs.oracle.com/javase/7/docs/api/java/util/Set.html) right underneath add() there is addAll(). I believe that is easier than doing a loop.
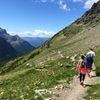
Stephen Wall
Courses Plus Student 27,294 PointsFirst you want to be able to add a tag to the set mTags:
public void addTag(String tag) {
// TODO: add the tag
mTags.add(tag);
}
Then you want to add a list of tags to the set mTags. Here I looped through the list and added them just as above:
public void addTags(List<String> tags) {
// TODO: add all the tags passed in
for (String tag : tags) {
mTags.add(tag);
}
}