Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial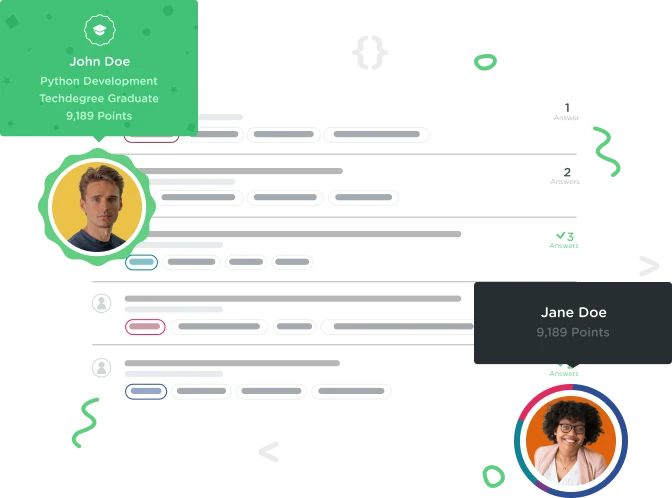

David Milburn
437 PointsI am stuck on the knock knock task
Hi I am stuck on this task can anyone help? It is taking too long to run so I guess I have created some sort of infinite loop??
Here is the code I am running:
boolean jokeNames; String who; do { // Person A asks: console.printf("Knock Knock.\n");
// Person B asks and Person A's response is stored in the String who: who = console.readLine("Who's there? "); jokeNames = (who.equalsIgnoreCase("orange") || who.equalsIgnoreCase("banana")); // Person B responds: if (jokeNames) { console.printf("%s who?\n", who); } } while (jokeNames);
/* So the age old knock knock joke goes like this:
Person A: Knock Knock.
Person B: Who's there?
Person A: Banana
Person B: Banana who?
...as long as Person A has answered Banana the above repeats endlessly
...assuming the person answers Orange we'd see
Person B: Orange who?
...and then the punchline.
Person A: Orange you glad I didn't say Banana again?
(It's a really bad joke that makes it sound like "Aren't you glad I didn't say Banana again?")
Let's just assume the only two words passed in from the console from Person B are either banana or orange.
*/
// ====BEGIN PROMPTING CODE====
boolean jokeNames;
String who;
do {
// Person A asks:
console.printf("Knock Knock.\n");
// Person B asks and Person A's response is stored in the String who:
who = console.readLine("Who's there? ");
jokeNames = (who.equals("orange") ||
who.equals("banana"));
// Person B responds:
if (jokeNames) {
console.printf("%s who?\n", who);
}
} while (jokeNames);
// ==== END PROMPTING CODE ====
2 Answers

Daniel Cunningham
21,109 PointsYour logic currently makes jokeNames equal to true if who equals "orange" or "banana". Because it will be true in both situations, the code will keep repeating itself for both instances.
Change jokeNames to:
jokeNames = (who.equalsIgnoreCase("banana")) // you should probably use .equalsIgnoreCase (rather than .equals) to make sure that case variants of "banana" are read as the same value. Additionally, You do not need the 'if' statement on jokeNames because it should print the variable who regardless of what is contained within it.
While you havent reached the punchline in the challenge yet, make sure you put the string variable 'who' into it rather than just writing the word "Orange". It failed when I did that :-P
Good luck!

MUZ140265 Blessing Kabasa
4,996 PointsFor the first stage you do the following
String who;
do {
console.printf("Knock Knock.\n");
who = console.readLine("Who's there? ");
console.printf("%s who?\n", who);
} while (who.equalsIgnoreCase("banana"));
for the second stage you then use the if statement
if (who.equalsIgnoreCase("orange")){
console.printf("%s I am glad you are not another Banana", who)};
David Milburn
437 PointsDavid Milburn
437 PointsThank you very much Daniel that has cleared things up for me! I have also completed the punchline as well... for the life of me I could not see my errors, this is a first for me but I am having great fun with programming. Have you done programming before you seem to know what you are talking about?
Also is the if statement not a mandatory part of a do, while loop? I was unsure whether or not this was required so I put one in but after reading this I have took it out and all seems good?
Thanks mate!
Daniel Cunningham
21,109 PointsDaniel Cunningham
21,109 PointsYou're welcome! I only had about 2 months of experience prior to joining Treehouse, so this service has been great in getting exposure to a bunch of different languages. I had only completed the Java Basics course about an hour before I caught the forum post (so it must have been good timing!)
For the do, while loop, think about the "while" as an alternative to "if". As long as the while loop remains true, the do event continues. The IF statement that we learned is good for a one time check. The way you placed it within the "do while" loop would have caused it to repeat the if statement in every iteration. In other languages, they also have looping statements like "unless" or "until", so I'm sure those will be covered in some future Java course.
If Java is your first language and gets difficult to understand, I'd recommend getting some exposure to some other languages. You begin to see very familiar concepts get repeated as well as different syntax options on how to pursue them. It's helped me catch on to things that were otherwise blocking me from continuing.
Best of luck!