Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial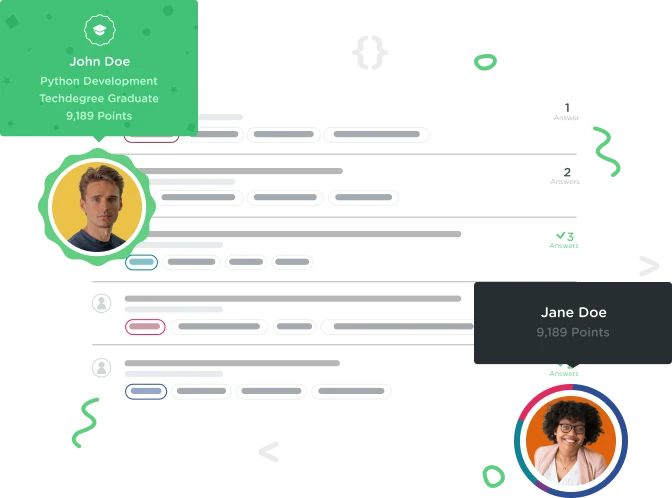

Samuel Piecz
8,143 PointsI am stuck on Challenge 1 of Python Collections.
Hello fellow Treehousers,
I am stuck on Challenge 1 of Python Collections.
The Challenge's instructions are as follows:
"OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end. I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you!"
My code is here.
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u"]
for letter in word:
if letter in vowels:
word.remove(letter)
return word
Thanks a bunch in advance for the help!
15 Answers

Umesh Ravji
42,386 Pointshttps://teamtreehouse.com/community/stage:11592
The other ideas from other topics:
- Using string concatenation to build a new string each iteration of the loop.
- Converting the input string to a list, then using remove. Then converting back to string.
- Creating an empty list, then appending characters to it each iteration of the loop. Then converting back to string.
You just cannot use remove on a string :)

Dave Laffan
4,604 PointsIf it helps, I used
def disemvowel(word):
vowels = "aeiouAEIOU"
new_word = []
for letter in word:
if letter not in vowels:
new_word.append(letter)
new_word = ''.join(new_word)
return new_word
which passed. However, why can't I use
for letter in word.lower():
and restrict the vowel string to just lowercase vowels? It works in PyCharm, but not for this challenge?

Umesh Ravji
42,386 PointsI'm going to assume some of the tests involve uppercase characters, which are ending up lowercase in the resulting string you are returning.
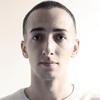
iansietro
11,361 PointsYou can use lower() while returning original lower/upper. Via solution below:
vowels = ['a','e','i','o','u']
def disemvowel(word):
result = []
for letter in list(word):
if not letter.lower() in vowels:
result += letter
result = ''.join(result)
return result

Harris Kunwar
Courses Plus Student 908 PointsWhy you can't use just lowercase vowels?
Say your word is AlphaBeta if you use word.lower, The result after the execution will be:
lphbt
But the actual result must be:
lphBt
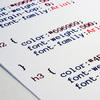
M Khan
7,024 PointsThat's brilliant

Dave Laffan
4,604 PointsSorry Umesh, don't quite understand what you are saying? If uppercase chars are involved, the test will pass so long as the vowels string contains both upper and lower to check against. However, in my efforts to make the code better, I replaced the for.... line with the single line of code above. I figured, by converting all to lower before any checks, this would negate the need to check for upper.
In PyCharm, this worked, and allowed me to replace the vowels string with just the lowercase vowels, but this wasn't accepted by the Treehouse challenge?
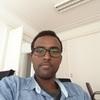
Mubarig CISMAN
Python Web Development Techdegree Student 2,547 PointsHi Benjamin, If you are still stuck in the Challenge, This should work for you.
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u"]
vowels = [element.lower() for element in vowels]
new_list = []
for letter in list(word):
if not letter.lower() in vowels:
new_list += letter
new_list = ''.join(new_list)
return new_list
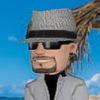
Thomas Beaudry
29,084 PointsNicely done Mubarig CISMAN, it took me awhilw to get this one!

RUIBO LIU
8,051 Pointsvowels = [element.lower() for element in vowels]
What's this line actually doing?
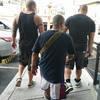
Omar G.
3,620 PointsHello, Thank you so much for this I just have a few questions. for letter in list(word): >>for this part why did you use list(word) not just word if not letter.lower() in vowels:>> I don't quite understand what this statement means. I think it means if the lower case of the letter is not a vowel added it to the new empty list you made before. Is that correct? Thank you for this. I got a better understanding of the exercise but I want to make sure I understand every part of the code as I found this challenge to be very difficult for me.
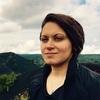
Manuela Sabatino
9,515 PointsThat is my answer, I hope it can help you out:
def disemvowel(word):
vowels = ("a", "e", "i", "o","u", "A", "E", "I", "O", "U")
for vowel in word:
if vowel in vowels:
try:
word = word.replace(vowel, "")
except ValueError:
pass
return word

Daniel Escobar
2,580 PointsManuela Sabatino, why parenthesis when you are creating a list? I tried the same code but with square brackets and my code does not work:
def disemvowel(word): vowels = ['a', 'e', 'i', 'o', 'u'] for letter in word: if letter in vowels: try: word = word.replace(letter, '' ) except ValueError: pass print(word)
disemvowel("Carlos")
this is my code. Any help would be appreciated
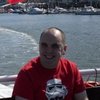
Sean Flanagan
33,235 PointsThank you Manuela Sabatino.
Let me see if I understand correctly.
vowels = ("a", "e", "i", "o","u", "A", "E", "I", "O", "U")
So this line lists all the vowels, in lower case then upper case?
The for loop creates a new variable called vowel and in that loop is an if statement. If there's a vowel in the word, go to the try block. that vowel will be replaced by an empty string in the word? In the case of a valueError, pass
, which means nothing happens?

Umesh Ravji
42,386 PointsStrings are not mutable in python, so you cannot remove from them. May I ask how the challenge is bugged? It appears to be passable as long as one includes capitals and lowercase vowels.

Samuel Piecz
8,143 PointsThanks for the comment Umesh! It is bugged. What would be the correct way to write the code? Is it possible just by using remove?
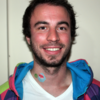
Peter Javorkai
31,477 PointsI'm a newbie to Python, and I'm also having some issues solving this challenge. Do you have a correct example to solve it?

Samuel Piecz
8,143 PointsHi Peter. If you post your code I can definitely take a look at it and see where you're getting caught up.
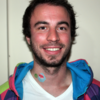
Peter Javorkai
31,477 PointsThanks, for the quick answer Samuel Piecz my code is the following:
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u"]
word = letters[]
try:
letters[] - ([vowels].upper() + [vowels])
else:
pass
", ".join(letters) = word
return word

Samuel Piecz
8,143 PointsHi Peter. My greatest apologies for such a late response. I got sidetracked.. Here is how I ended up solving the challenge. Best of luck!
vowels = ["a", "e", "i", "o", "u"]
word = "pizza"
empty_string = ""
for letter in word:
if letter not in vowels:
empty_string += letter
print(empty_string)
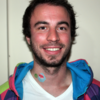
Peter Javorkai
31,477 PointsThanks Samuel, for some reason it's not accepting for me, but I'll keep trying! :/

Samuel Piecz
8,143 PointsHi Peter. You can't copy and paste it if that is what you're doing. It must be reformatted for the function that the challenge asks for. The answer should help ;)
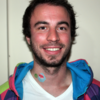
Peter Javorkai
31,477 PointsThank you Dave Laffan and Umesh Ravji for the help, it's amazing how active this community is. I'm amazed and better understood the issue.

Umesh Ravji
42,386 PointsHi Dave Laffan, while the code does work in PyCharm, it doesn't produce the same result. For example: disemvowel('BANANA')
results in BNN
. Where as, if you use word.lower()
, your result becomes bnn
(which is not the correct answer.

Dave Laffan
4,604 PointsAh yes, thank you. Simple, when you put it like that
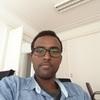
Mubarig CISMAN
Python Web Development Techdegree Student 2,547 PointsIn order to lower case the vowels, we can go through the list in one go
vowels = ["a", "e", "i", "o", "u"]
vowels = [element.lower() for element in vowels]
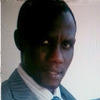
BENJAMIN UWIMANA
Courses Plus Student 22,636 PointsThanks Mubarig. I got it. Thanks a lot.

Tucker Weston
Courses Plus Student 13,128 Pointsdef disemvowel(word): vowels = ['a','e','i','o','u']
string_vowels = ''.join(vowels)
upper_vowels = list(string_vowels.upper())
vowels += upper_vowels
word_list = list(word)
for vowel in vowels:
for letter in word_list:
if vowel == letter:
word_list.remove(letter)
word = ''.join(word_list)
return word
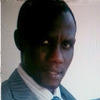
BENJAMIN UWIMANA
Courses Plus Student 22,636 Pointsdef disemvowel2(word): vowels = ["a","e","i","o","u"] my_list = list(word) word = "" for letter in my_list: if letter.lower() not in vowels: word += letter print(word) return word
disemvowel2("BEnjamin")
Samuel Piecz
8,143 PointsSamuel Piecz
8,143 PointsThanks Umesh! I will try and use these methods.
** Updated **
Here is the method I found to work. Didn't try the other ones, but am happy to have heard multiple solutions for the problem thanks to Umesh.