Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial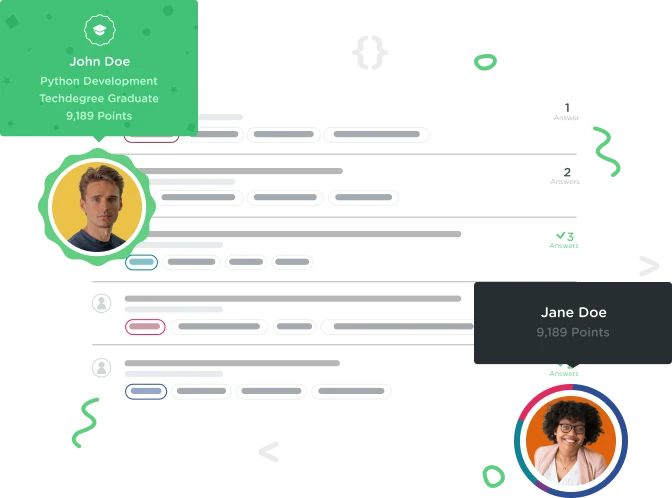

Larab Mohsin
Courses Plus Student 262 PointsI am not sure how to do this? Can someone give me a detailed explanation please?
This challenge
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
this.tiles += tile;
}
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
return false;
}
}
2 Answers
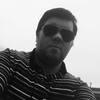
Stephen Kilgore
Java Web Development Techdegree Student 2,610 PointsI'm adding a new answer for ease of understanding.
You are close, and you could use a conditional if you want, but it isn't necessary.
You can just return the expression which will automatically evaluate to either 1 or 0.
Example:
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
return tiles.indexOf(tile) != -1;
}
}
I'll show you a few ways to solve with a conditional if statement. This is not the best way. The most concise way is to just return the result of the expression.
The challenge won't let you solve with a conditional if statement, but if it did, here's how it would look:
public boolean hasTile(char tile) {
if (tiles.indexOf(tile) >= 0)
{
return true;
}
return false;
}
}
On the above one, we don't need an else statement because code execution stops after return is ran. If we get to return false, we automatically know that it is false for this reason, because if it were true execution would have stopped within the conditional.
Here's a third way that's a bit interesting. It's a bit of a round about way. It's called the ternary operator, and it's awesome. It's a way to assign something to a variable based on a conditional, in one line!
public boolean hasTile(char tile) {
boolean ternaryFun = tiles.indexOf(tile) >= 0 ? true : false;
return ternaryFun;
}
}
the format is:
condition ? value if condition returns true : value if condition returns false;
read more about it at:
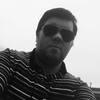
Stephen Kilgore
Java Web Development Techdegree Student 2,610 PointsBasically, this challenge stores a list of the tiles that a player has (remember, this is scrabble, so each tile is a letter) into a string. Let's say I have the tiles a, b, c, and u in my hand. the string that represents that would be "abcu" and it would be stored into the 'tiles' field.
All this challenge is saying is that the addTile method, when called, should add a new tile to the players hand. Let's say tiles equals my above example, "abcu". if I call addTile('x'), the new value that addTile() should store for the tiles field is "abcux" You've just learned how to join two strings together, so all you need to do is add the character that was passed into addTile as an argument onto the end of the tiles String.
I hope this is clear!
EDIT:
For hasTile, we just need to check if the character occurs at least once in the string. To do that, we need to use the indexOf method.
See the following:
https://www.tutorialspoint.com/java/java_string_indexof.htm
So, the indexOf method returns an integer that is the index (or position) of a character in a String. if the character is not present in the String, it returns -1.
So, you need to make do a conditional check to see if if the char tile is in the String tiles by using that indexOf method. If it is, return true. If it isn't, return false.

Larab Mohsin
Courses Plus Student 262 PointsI am sorry I meant that I need help with the "hasTile" method!

Larab Mohsin
Courses Plus Student 262 PointsYes, I know what indexOf does. I tried this before:
boolean isTile = tiles.indexOf(tile) != -1 if(isTile){ return true }else{ return false }
didn't work.... help haha
Stephen Kilgore
Java Web Development Techdegree Student 2,610 PointsStephen Kilgore
Java Web Development Techdegree Student 2,610 PointsSorry about that, I updated my answer.