Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial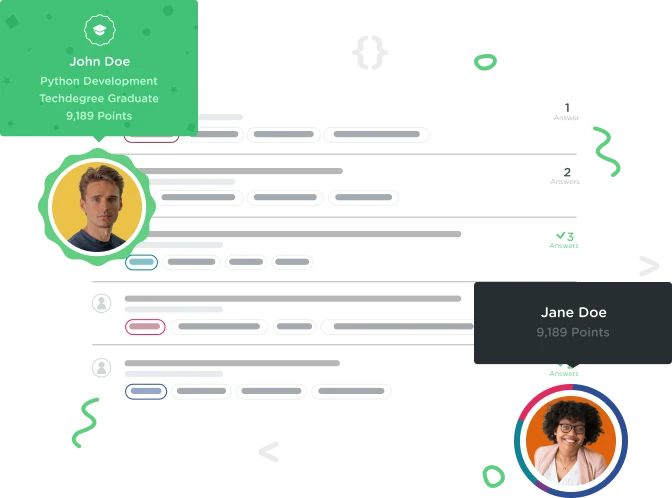

Jiten Mistry
4,698 PointsI am completely stuck on this question
i think i dont understand the logic behind what is being asked
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
1 Answer
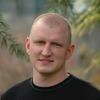
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsSo when coming into a challenge, dont just read the code to figure out which parts are broken and need fixing. Think about what is SUPPOSE to be happening. Order or steps things would happen in, what should be returned. I take just a minute to look at each class file, even though it says you dont need to change anything it is still good to look at its structure.
Customer.java All that makes up a Customer is a single class member variable mName and a "getter" to get that specific customer's name.
CustomerSupportRep.java Has quite a bit more to it. A Customer Support Representative also has a class variable mName. But it also stores a List<Customer> named mAssistedCustomers, which I read it as "a List of Customer objects". This way anytime we refer to a specific Customer Support Representative we can get a list of every Customer they have assisted already.
CallCenter.java This is what they want you to build based off the other two. So CallCenter holds "a Queue of CustomerSupportRep objects". This sounds right, a call center would have a ton of Support Reps answering phones. It has 2 methods, both which return nothing ( public void ). But 1 of them ( public void acceptCustomer ) takes a parameter which "must be of the type Customer. Cant be some String or Integer when it GOES IN to the function.
Okay, so what do I need to do. The comments are there to help and they all are inside the method acceptCustomer.
Here is a very broken down thought process I try to go through when code is just beating my brain up.
TODO 1 Wait until there is an available rep. How do I know when a rep IS available? Well my CallCenter holds a Queue. Any "Rep" in this Queue is technically WAITING to answer a call, they are ready so I could grab the first rep available in that Queue. What is a Queue and what can IT do? Queue Doc
So this variable mSupportReps inside my CallCenter is "Of Type Queue which holds my available CustomerSupportReps" so I can call these methods it inherits. Methods like .add() which let me add an item into this Queue or something like .poll() which wont just remove but it also returns the item it removes that way you could store it in a new variable lets say like a CustomerSupportRep csr; variable HINT HINT. ;)
So when acceptCustomer is called I really need to ensure that before a Customer is truly accepted we check some conditions first. so while a certain condition isnt true like having an empty or null return in my Queue I am going to call playHoldMusic(); but if that statement is false it will never enter a loop. It will skip it completely and move on to
Does this help at all? If not let me know I will try another approach.
Jiten Mistry
4,698 PointsJiten Mistry
4,698 PointsHi Chris, Thank you for that it helped me understand it more! This is what I've got so far
" while(!(mSupportReps.poll() == null || mSupportReps.isEmpty())) { playHoldMusic(); } csr = mSupportReps.poll(); csr.assist(customer); mSupportReps.add(csr); "
but having no luck. Could you please guide me in the right direction please?
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsChris Howell
Python Web Development Techdegree Graduate 49,702 PointsYou have the right idea. But you dont want to use mSupportReps.poll() just YET. Remember .poll() as it says in the docs "Method Summary" section. poll() "retrieves AND removes from the queue as soon as you call it. So instead you just want to PEEK inside the queue FIRST to see if anything is there but leave it there.
So you call .poll(). Then it says "HEY I FOUND SOMETHING, this (CustomerSupportRep) here I took it out of the Queue now its yours to have. It was the only thing in there so if you call .poll() if there were more the Queue would shift just like the checkout line at a grocery store.
if you use .peek() for your while loop. Then you are basically walking around the outside of the checkout line looking for the person about to checkout.
Also when you call .peek() IF nothing is there, it checks for if its empty IF its true(empty) then it instead of CustomerSupportRep it gives you null back. So you only have to check for a null.