Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial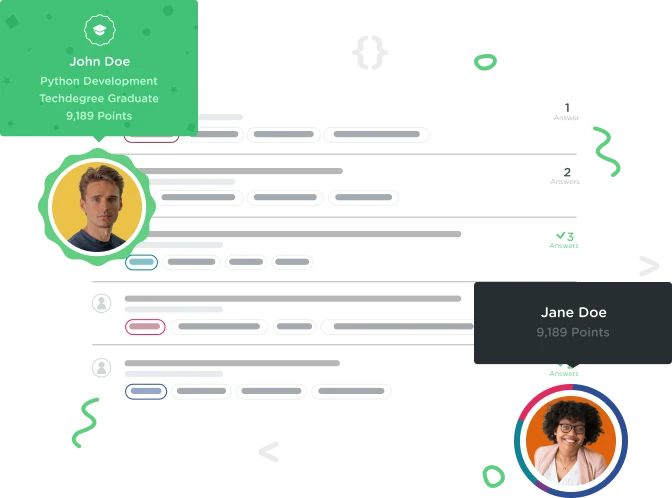

tomtrnka
9,780 PointsHow to write a method for printing all items from Lists AND Sets?
I was trying out some things with lists and sets, and i got tired of copying multiple rows of code, just so I could see the content of lists/sets printed in console. (im not using repl, using IntelliJ Idea).
I got some ArrayLists, HashSets and TreeSets - all with generic type of String.
I wanted to write a single method, which would take any list or any set as a parameter. This method would print out all the strings inside arrayList/hashSet/treeSet,
At the beginning, I wrote these 2 methods:
public void printList(List nameOfSetOrList) {
for (Object language : nameOfSetOrList) {
System.out.println(language);
}
}
public void printSet(Set nameOfSetOrList) {
for (Object language : nameOfSetOrList) {
System.out.println(language);
}
}
Then I merged them together like this:
public void printListOrSet(Object nameOfListOrSet) {
if (nameOfListOrSet instanceof List) {
List<String> myList = (List) nameOfListOrSet;
for (String list : myList) {
System.out.println(list);
}
} else if (nameOfListOrSet instanceof Set) {
Set<String> mySet = (Set) nameOfListOrSet;
for (String set : mySet) {
System.out.println(set);
}
}
}
Any idea how to rewrite this mess so its simpler and cleaner?
Thank you
3 Answers

Seth Kroger
56,415 PointsBoth List and Set are both part of the Collections Framework. Part of that means that they both descend from the same superclass, Collection. Perhaps you could use that fact to clean things up?

markmneimneh
14,132 PointsI would check the switch statement
https://docs.oracle.com/javase/tutorial/java/nutsandbolts/switch.html
Hope this helps ... if this answers your question, please mark the question as answered.
Thanks

Tomas Verblya
1,998 PointsCould not quote the post I wanted to and I'm not sure how to format the code, cause the cheatsheet doesn't open :( . But I'd like to understand the part here :
public void printListOrSet(Collection nameOfListOrSet) { for (Object list : nameOfListOrSet) { System.out.println(list); } }
OBJECT list. Why is this an object? Why not a Collection?

Seth Kroger
56,415 PointsThe code is iterating through all the items of the Collection. So we're dealing with individual items, not collections. Since the generic type was left unspecified (i.e., there is no <ClassName>
after Collection) we use Object.
tomtrnka
9,780 Pointstomtrnka
9,780 PointsThank you! Youre absolutely right!
Works fine :-)