Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial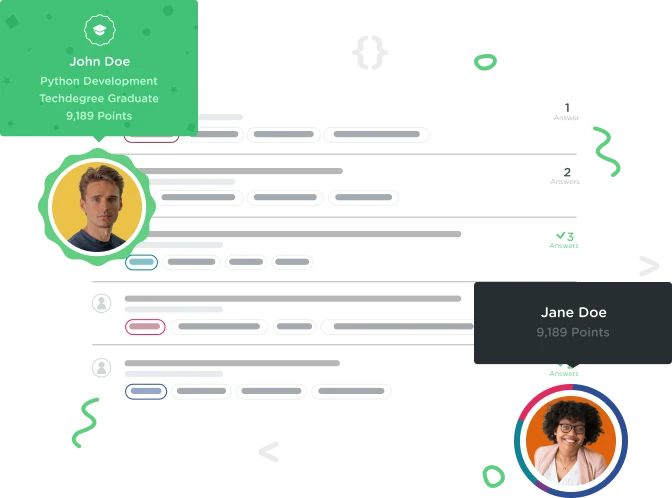
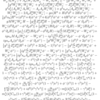
Matthew Alexander
14,000 PointsHow to solve this problem?
Can you help me solve this:
I've added a new class called Blog. It is initialized with a list of BlogPost objects. Create a method in the Blog class called getAllAuthors that loops over all the posts and returns a java.util.Set of all the authors, which are stored as Strings. They should be sorted alphabetically.
Thank you for your help. If you don't mind can you explain your code.
3 Answers
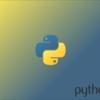
Kevin Faust
15,353 PointsHey Matthew,
Let's go through the first thing. Let's first construct what is given to us in the instructions. We know that we want to create a method that brings back a set of the authors. The author names are all Strings:
public Set<String> getAllAuthors() {
//code here
return null;
}
Inside the method, we first need to initialize a Set so we can add things to it. Since we want the authors to be in alphabetical order, we use a TreeSet.
Set <String> set = new TreeSet<>();
So now we got our empty Set in place. Now we want to add all the authors name right? We use the for-each loop to do this. In our mPosts list, we will loop through each one and grab the author's name. Once we grab the author's name, we add it to the set
for (BlogPost authorName : mPosts) {
set.add(authorName.getAuthor());
}
As you can see, since the list is made up of BlogPosts, that is the data type that we will be using. Im sure you already know know the for each loop works.
The Set interface has a method called add and we simply call the getAuthor() method and add it to our set each time the loop runs
All that's left now is to return the set. In case you want the whole thing, I attached it below for reference
public Set<String> getAllAuthors() {
Set <String> set = new TreeSet<>();
for (BlogPost authorName : mPosts) {
set.add(authorName.getAuthor());
}
return set;
}
Happy coding and good luck ,
Kevin
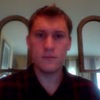
Casey Huckel
Courses Plus Student 4,257 PointsThanks Kevin, I got it!
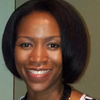
Natasha Johnson
12,285 PointsKevin F, EXCELLET EXPLANATION!! I did have to go back and import TreeSet. Thanks!
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsGreat answer as always, Kevin !!!!
Matthew Alexander
14,000 PointsMatthew Alexander
14,000 PointsThank you so much Kevin for your help. However I have a question, why do we use the add method for a TreeSet? Why don't we use the addAll method like in the example that Craig gave us? Can a Set use an add method?
I am sorry for my foolish questions.
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsHey Matthew,
We can think of it like this.
When we use this method:
authorName.getAuthor()
What does this return? It returns a single author name aka a single string right? Since we are only adding one thing, we use the add() method. We are not adding multiple things but rather just a single thing. The addAll() method is used when you want to add something which contains stuff inside of that. Think of something like a list. A list is something which contains stuff inside of that right? That's when we would use the addAll() method.
I'm not one that particularly likes the java docs as it's not beginner friendly and quite frankly, it's just plain ugly
But I do use it to search up what methods something can use.
Edit august 2016: i dont really use java nowadays but after lots of coding exposure, the java docs are not as bad as they were to me in the past. For those that struggle, just trust time and patience
Here you can see all the methods that we can use with the Set and you can see add() is part of it!
https://docs.oracle.com/javase/7/docs/api/java/util/Set.html#addAll(java.util.Collection)
Kevin
Casey Huckel
Courses Plus Student 4,257 PointsCasey Huckel
Courses Plus Student 4,257 PointsKevin, I've got this compiler error when I tried your solution:
./com/example/Blog.java:8: error: cannot find symbol public Set getAllAuthors() { ^ symbol: class Set location: class Blog ./com/example/Blog.java:9: error: cannot find symbol Set set = new TreeSet<>(); ^ symbol: class Set location: class Blog ./com/example/Blog.java:9: error: cannot find symbol Set set = new TreeSet<>(); ^ symbol: class TreeSet location: class Blog Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. 3 errors
Kevin Faust
15,353 PointsKevin Faust
15,353 Pointshey casey, you forgot your imports
Casey Huckel
Courses Plus Student 4,257 PointsCasey Huckel
Courses Plus Student 4,257 PointsAs you suggested, I need to import, but import what? I see no instructions on what to import
Kevin Faust
15,353 PointsKevin Faust
15,353 Pointsjava.util.Set and java.util.TreeSet
Sets and treesets come from the collections framework and to use them you must import them. In the videos, I am definetely sure that you have been importing them and that craig has said you must import them to use them.
Bob Allan
10,900 PointsBob Allan
10,900 PointsKevin Faust , holy cow that is the best explanation of ANYTHING that I've seen on Treehouse. I just learned several skills, including how to properly answer a question about Java. THANK YOU!