Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial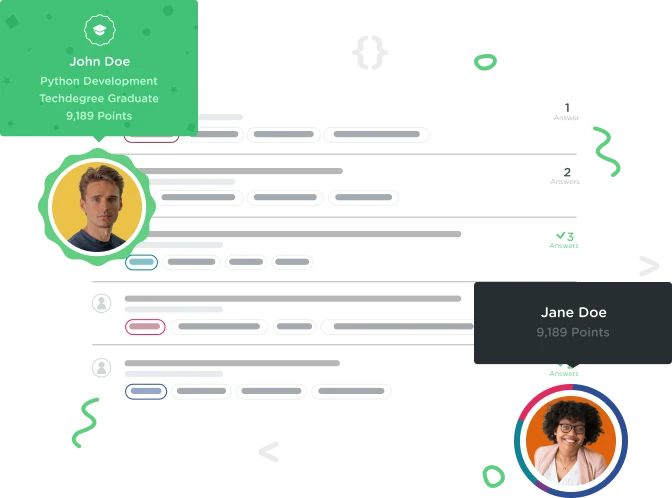
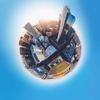
Joel Crouse
4,727 PointsHow to randomize a dictionary and display the keys and values
Example: I have a dictionary of songs where the key is the song title and the value is the artist.
let songs = ["Song Title": "Artist", "Song Title": "Artist", "Song Title": "Artist"]
In my view I have two labels, one for the song title and one for the artist and I want to populate each label with a song title and corresponding artist from the dictionary randomly after a user taps a button.
So far I've creating the IBOutlets for each label and an IBAction for the button so I just need to create a function that pulls out a random song from the dictionary and allows me to access the songs title (key) and artist (value). How could I do this?
2 Answers
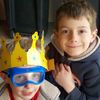
Chris Stromberg
Courses Plus Student 13,389 Pointslet musicLibrary = ["Comfortably Numb": "Pink Floyd", "Hells Bells": "AC DC", "Lonely Boy": "The Black Keys"]
func randomSongFrom( musicLibrary library: [String: String]) -> String {
// Gets song titles and places into an array, so that song titles can be picked randomly by index.
var songs = Array(library.keys)
// Generates random number to place for index value in song array.
let randomNumber = GKRandomSource.sharedRandom().nextInt(upperBound: songs.count)
let song = songs[randomNumber]
let artist = library[song]
return "Now playing \"\(song)\" by \(artist ?? "No Artist")."
}
randomSongFrom(musicLibrary: musicLibrary)
randomSongFrom(musicLibrary: musicLibrary)
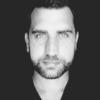
Jhoan Arango
14,575 PointsHello,
To extend on Chri's answer..
You may want to also do it this way since you mentioned that you want to populate some fields. Instead of returning just a string you could return a tuple with the title and artist information about the song.
import GameplayKit
// In order for you to be able to use GKRandomeSource you have to import GameplayKit
let library = ["Beat it": "Michael Jackson", "Like a Virgin": "Madonna", "Mine": "Bazzi"]
func randomSongFrom(_ library: [String: String]) -> (title: String, artist: String) {
let titles = Array(library.keys)
let randomIndex = GKRandomSource.sharedRandom().nextInt(upperBound: titles.count)
let title = titles[randomIndex]
let artist = library[title] ?? "Unknown"
return (title , artist)
}
// Getting the random song
let song = randomSongFrom(library)
// Breaking down the song
let title = song.title
let artist = song.artist
Good luck, hope you find this useful
Joel Crouse
4,727 PointsJoel Crouse
4,727 PointsWhy did you declare the songs variable from outside the function?
And why is the return statement formatted that way?
return "Now playing \"\(song)\" by \(artist ?? "No Artist")."