Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial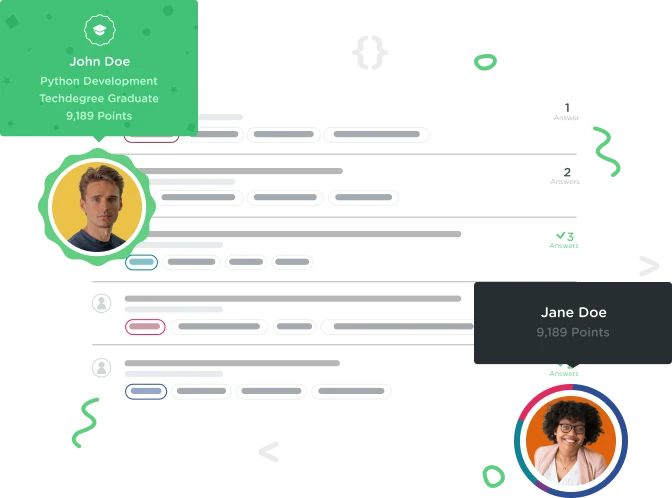

yahyaalshwaily2
Python Development Techdegree Graduate 20,049 PointsHow to raise an exception for num_of_people for when the input is a float and not an integer?
Hi,
I improvised and wrote elif num_of_people != int(): print("please enter a whole person") and ofcourse I't didn't work.
Help please :)
4 Answers
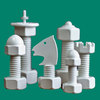
Steven Parker
241,957 PointsI assume you converted the input using "float()"? You'd need to for this to work.
But then the issue is that you forgot to pass the value to the "int" function:
elif num_of_people != int(num_of_people):
Of course, if you convert the string using "int" in the first place the system will give an error if the value is not integer.

yahyaalshwaily2
Python Development Techdegree Graduate 20,049 PointsMaybe it will help you to look at the entirety of the code.
import math
def split_check(total, num_of_people):
if num_of_people != int(num_of_people):
raise ValueError ("people cannot be fragmented")
if num_of_people <=1:
raise ValueError ("more than one persone is required to split the bill")
if total <=0:
raise ValueError ("Bill cannot be negative or zero")
return math.ceil (total / num_of_people)
try:
total_due = float(input("Please enter total "))
total_persons = int(input("For how many people? "))
amount_due = split_check(total_due,total_persons)
except ValueError as err:
print ("please enter a valid value")
print ("({})".format(err))
else: print("Each person owes ${}".format(amount_due))

yahyaalshwaily2
Python Development Techdegree Graduate 20,049 PointsSorry Steven I will re-do it with the markdown cheatsheet

yahyaalshwaily2
Python Development Techdegree Graduate 20,049 PointsHi Steven Parker, It took me some time to go through the markdown course :D but it turned out its just 3 back ticks -> python -> 3 back ticks :D.
anyway, here's the complete code looking nicer
import math
def split_check(total, num_of_people):
if num_of_people != int(num_of_people):
raise ValueError ("people cannot be fragmented")
if num_of_people <=1:
raise ValueError ("more than one persone is required to split the bill")
if total <=0:
raise ValueError ("Bill cannot be negative or zero")
return math.ceil (total / num_of_people)
try:
total_due = float(input("Please enter total "))
total_persons = int(input("For how many people? "))
amount_due = split_check(total_due,total_persons)
except ValueError as err:
print ("please enter a valid value")
print ("({})".format(err))
else:
print("Each person owes ${}".format(amount_due))

yahyaalshwaily2
Python Development Techdegree Graduate 20,049 Pointsagain, thank you a lot! :D
yahyaalshwaily2
Python Development Techdegree Graduate 20,049 Pointsyahyaalshwaily2
Python Development Techdegree Graduate 20,049 PointsHi Steven,
thank you very much for your input.
It seems that not passing the variable inside the int argument => int(num_of_people) was indeed an obvious mistake, but unfortunately it did not fix the issue, I still cannot make the error message for fractional people to pop up in the console, do you have any more suggestions?
my kind regards, Yahya.
Steven Parker
241,957 PointsSteven Parker
241,957 PointsPlease show the entire code. Be sure to use Markdown formatting (as I did above) to preserve the appearance.
Steven Parker
241,957 PointsSteven Parker
241,957 PointsI see that the conversion is being done with "int":
total_persons = int(input("For how many people? "))
So a non-integer value will cause a ValueError and be handled by the "except" before your test gets a chance to run.
But if you do the conversion with "float" instead of "int", it will.
yahyaalshwaily2
Python Development Techdegree Graduate 20,049 Pointsyahyaalshwaily2
Python Development Techdegree Graduate 20,049 PointsSteven Parker Whoa! that totally worked! You're awesome man, thank you for your help!!
I get it now, since we already expecting an integer input, the ValueError for invalid unit type will trigger instead. That's the mistake.
But if we have the
python num_of_people = float(input("number of people")
it can accept an integer so thepython if num_of_people != int(num_of_people)
will not activate if the "float" input is an integer but will activate if it is indeed actually a float input.