Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial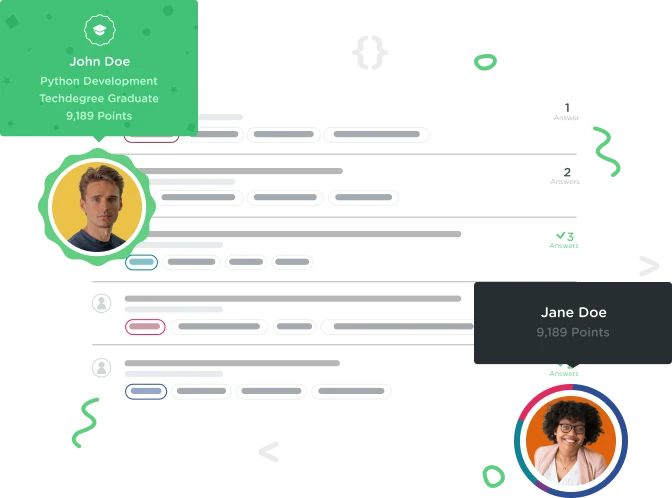

Brandon Wash
Courses Plus Student 1,186 PointsHow to properly use if statement
Add an if statement that checks to see if firstExample is equal to secondExample. If it is, print out "first is equal to second".
// I have imported a java.io.Console for you, it is named console.
String firstExample = "hello";
if (first
String secondExample = "hello";
String thirdExample = "HELLO";
2 Answers
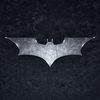
Logan R
22,989 PointsJava does not read Strings the same way it reads integers, doubles, floats, and so on. In an if statement where you are comparing an integer, you can simply use the ==.
if(5 == 1) {
// Do something
}
With strings however, you must use the method called equals() or equalsIgnoreCase(). So in this case:
// I have imported a java.io.Console for you, it is named console.
String firstExample = "hello";
String secondExample = "hello";
String thirdExample = "HELLO";
if(firstExample.equals(secondExample)) {
console.printf("first is equal to second");
}
The equals method checks every character and sees if they are equal. Hello and hello would not be equal.
The equalsIgnoreCase method only checks to see if every character is the same, regardless of case. Hello and hello would be equal.
I hope this helps out. If you still don't understand, feel free to leave a comment :)

Brandon Wash
Courses Plus Student 1,186 PointsThanks Craig I certainly appreciate the feedback. I am a early developer but very eager to learn. I want to code until I leave the planes of this Earth. I found it very interesting and it arouses my curiosity. Do you have any early advice for a young and determine developer such as myself?
Ricky Catron
13,023 PointsRicky Catron
13,023 PointsI addition to this great answer .equals() is used because Strings are objects. Objects are compared with the .equals method. == is used for primitive types.
primitive type example:
Object type example: Integer is a wrapper class for the primitive int type
String type example:
Goodluck, --Ricky
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherAlso remember to use the
equalsIgnoreCase
method on the string.