Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial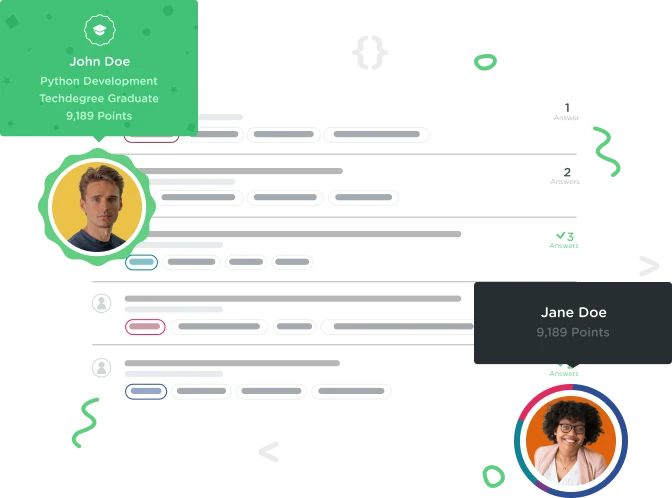
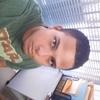
Kevin Fernandez-Gonzalez
16,790 Pointshow to normalize the dollar sign
this is my second try in getting help, I improve the code but i can find a way to deal with the dollar symbol. i would appreciate example and not the actual answer please and thank you.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
private String normalizeDiscountCode(String discountCode){
char code = discountCode.charAt(0);
if(!Character.isLetter(code) || code != '$' ){
throw new IllegalArgumentException("Invalid discount");
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
2 Answers

andren
28,558 PointsYour condition for dealing with the $ sign is fine, but remember what the if statement should do. It should trigger if the character is not a letter and it is not the $ sign. Your current if statement does something slightly different, which has a pretty big effect on how it functions.
I'll also point out that the challenge is to check if any character in the discount code is invalid, currently you are only testing the first letter of the discount code, which won't enable you to complete the challenge.
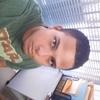
Kevin Fernandez-Gonzalez
16,790 Pointsthanks you so much it really help and i got me to under stand more
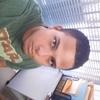
Kevin Fernandez-Gonzalez
16,790 PointsI did with the for loop and nest inside the if and it work, thanks for your help
Kevin Fernandez-Gonzalez
16,790 PointsKevin Fernandez-Gonzalez
16,790 Pointsyes is true, but what other way is there to check each character? do i use indexOf() instead?
andren
28,558 Pointsandren
28,558 PointsI would personally use a loop, I don't know what loops have been thought in the Java course at the point you are at, but if you know how "for" loops work then that would work pretty well here. Even if you only know "while" loops (which is the most basic loop type) you should be able to cobble together a loop that runs once for each letter in the string and checks said letter within the loop.
Edit:
I just went and experimented a bit and it's definitively possible to solve the challenge using a while loop, if you end up stuck then I have posted the way to do it here. I have intentionally left the mistake you have in you if statement in my solution, so if you do end up looking at it you can see how to loop though the characters but you will still have to fix the if statement yourself.
Do note that looping through it using a while loop is not be best way, and I only choose to test that because that is the only loop type I know they teach early on in the Java course. Ideally you would use a for loop for a task like this, but I don't remember if you have been thought how to use those in the course.