Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial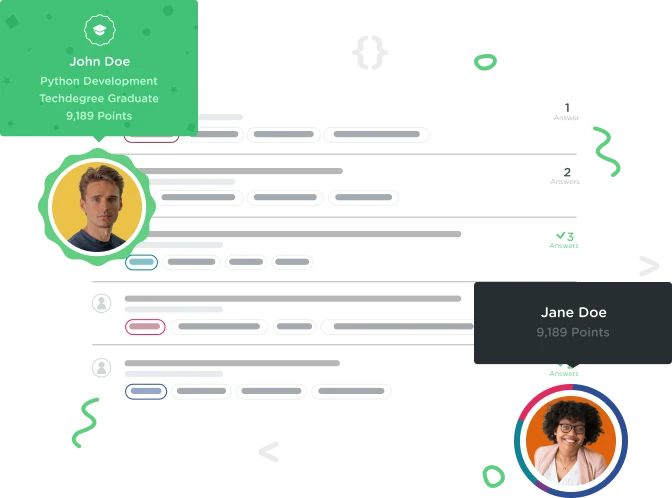

Miika Vuorio
3,579 PointsHow to make an area instead of a point?
I'm trying to do the extra credit which says to try and make it so that the flies can not spawn on top the player. I know how I would program it, but I don't know how to create an area in unity
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FlySpawner : MonoBehaviour {
[SerializeField]
private GameObject flyPrefab;
[SerializeField]
private GameObject player;
[SerializeField]
private int totalFlyMinimun = 12;
[SerializeField]
private float spawnArea = 25f;
private Vector3 nonSpawnArea;
public static int totalFlies;
// Use this for initialization
void Start () {
totalFlies = 0;
}
// Update is called once per frame
void Update () {
// While the total number of flies is less than the minimum...
while (totalFlies < totalFlyMinimun) {
// ...then increment the total number of flies
totalFlies++;
// ...create the area in which the flies can not spawn in
nonSpawnArea = new Vector3 (player.transform.position.x + 3f, 2f, player.transform.position.z + 3f);
// ...create a random position for a fly...
float positionX = Random.Range(-spawnArea, spawnArea);
float positionZ = Random.Range(-spawnArea, spawnArea);
Vector3 flyPosition = new Vector3 (positionX, 2f, positionZ);
// ...check that the fly isn't spawning on top of the player
if (flyPosition != nonSpawnArea) {
// ...and create a new fly
Instantiate(flyPrefab, flyPosition, Quaternion.identity);
}
}
}
}
I know what's the problem. The nonSpawnArea is not a square, but a point. Also the if statement has to check if it's in the nonSpawnArea not if it isn't it. Also if there would be a better way of doing it please inform me.