Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial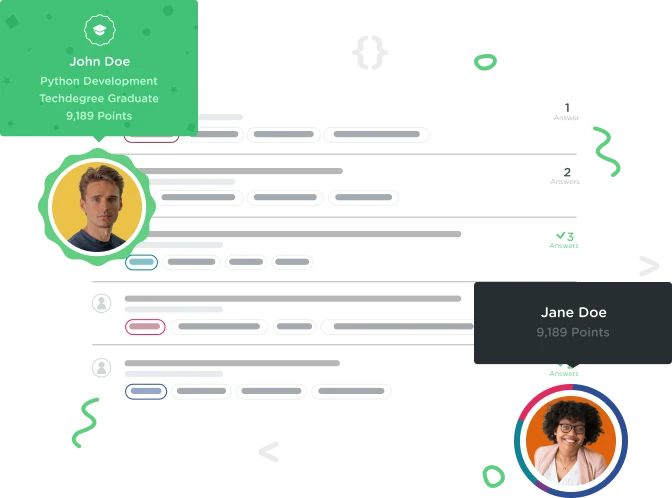
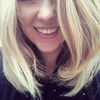
Agnete Djupvik
1,281 PointsHow to loop through posts and their categories?
I'm not sure as to how to access all categories within all the blogposts. I don't know how to loop through the objects. How can I do this?
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.TreeSet;
import java.util.Set;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
public Map<String, Integer> getCategoryCounts(){
Map<String, Integer> count = new HashMap<String, Integer>();
for(String
List<String> categories =
return count;
}
}
2 Answers
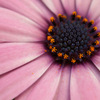
Yael P.
2,816 PointsHi Agnete,
I will try to explain what I did, step by step:
First of all, I've added a new method under Blog.java, as you did.
The challange asked to add a Map, and return the category and a count, so Map<String, Integer> (Integer as an object), as you did.
Next, I've decleared new object, as you did.
Now, we need to loop over all posts, so I've added For statment:
for (BlogPost post : mPosts)
It's moving all over the posts (mPosts), similar to previous challange.
Now I want to get the category of each post (each post can have only 1 category) so
String category = posts.getCategory();
Now I want to add the count, and make sure that it's not null, and if not, add to the counter:
Integer count = countMapOfCategory.get(category);
if (count == null) {
count = 0;
}
count ++;
Then, put the values
countMapOfCategory.put(category, count);
and return
return countMapOfCategory;
The whole code in one piece:
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> countMapOfCategory = new HashMap<String, Integer>();
for (BlogPost post : mPosts) { //getting all the posts
String category = post.getCategory();
Integer count = countMapOfCategory.get(category);
if (count == null) {
count = 0;
}
count ++;
countMapOfCategory.put(category, count);
}
return countMapOfCategory;
}
Hope that I've helped. If you got any questions, let me know.
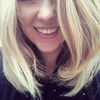
Agnete Djupvik
1,281 PointsCool! That really helped. Thanks!
Lee Reynolds Jr.
5,160 PointsLee Reynolds Jr.
5,160 PointsVery nice job breaking it down like that. It helped me out a lot :)