Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial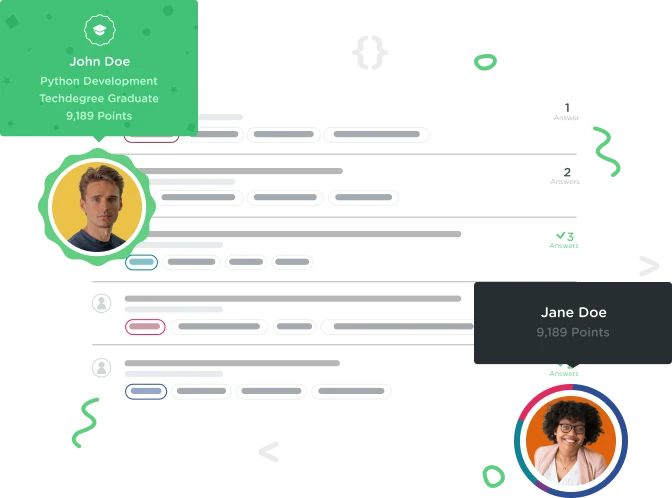

Logan Cornelius
16,876 PointsHow to initialize a property in a subclass that is unique from it's superclass?
class Roommate {
let name: String
var age: Int
var skills: [String]
var sayings: [String:String]
init(name:String, age:Int, skills: [String], sayings: [String:String]) {
self.name = name
self.age = age
self.skills = skills
self.sayings = sayings
}
}
class Pet: Roommate {
let isAnimal: Bool = true
var ownerIs: String //how do I initialize this since it is unique from the superclass
override init(name: String, age: Int, skills: [String], sayings: [String : String]) {
super.init(name: name, age: age, skills: skills, sayings: sayings)
}
}
2 Answers
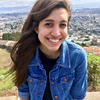
Sarah Hurtgen
Treehouse Project ReviewerAre you wanting to have an initializer for "Pet" that allows you to fill in who the "ownerIs" as well as the original "name", "age", etc? One thing you could try is removing the word "override", and add the parameter "ownerIs: String" into the init().
init(name:String, age:Int, skills: [String], sayings: [String:String], ownerIs: String)
With this, I think you create a unique initializer that is specific to "Pet". In the body you would leave what you have already (the super.init), and would assign "ownerIs" to self, like you did for the variables in your "Roommate" init.
I'm not sure if that is quite what you were looking for, but I hope it helps!

Logan Cornelius
16,876 PointsAwesome! well thanks so much! I appreciate the help!
Logan Cornelius
16,876 PointsLogan Cornelius
16,876 PointsOk! This is working now! thank you!
class Pet: Roommate {
}
So I have to incluce the unique property in the init, and set it equal to self, but then I don't include it in the super.init initializer because it is not found in the super class. That makes sense!
why do I take the override out fully since most of the properties are still being overridden?
Sarah Hurtgen
Treehouse Project ReviewerSarah Hurtgen
Treehouse Project ReviewerHonestly, I'm pretty new to trying to understand classes/subclasses, so I'm not completely sure- but I think it's because instead of overriding the parent class init, you're adding a unique one. If you backspace the "ownerIs" parameter (leaving you with the inherited init), it will pop up that you need to need to use the word "override", but once you add a whole new parameter, it is a now a different init.
For example, if you weren't adding any new parameters and just wanted to override the init to do something different, you could do something like this:
So now when that original init is used, it does the added function of printing "Hello!"
There may be a way to override the parent init and actually change the parameters, but at this point, it is beyond my knowledge :)