Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial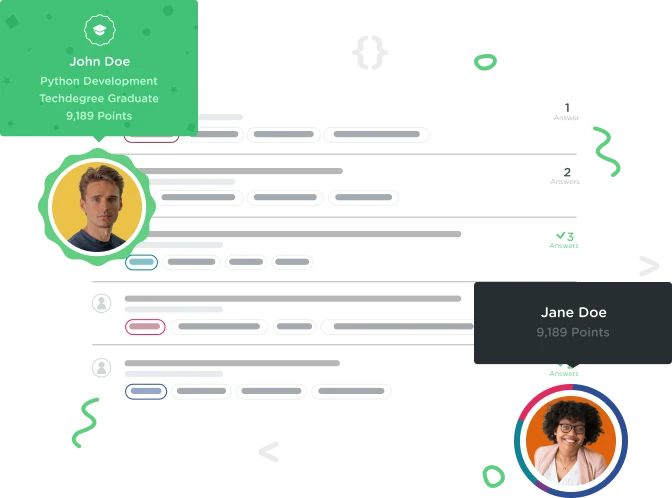

Inge L
30,058 PointsHow to add the styles(JavaFX)?
Using the Brand enum, I'd like add styles to the root container. I added an fx:id for the container and I wrote some code to remove the brand styles.
Can you please add the styles for the Brand in the switchBrand method? Please call the clearBrandStyles method first.
package com.example;
import com.example.model.Brand;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
public class Controller {
@FXML
private VBox container;
public void handlePlay(ActionEvent actionEvent) {
System.out.println("[MUSIC PLAYING] - I shot the sheriff, but I didn't shoot the deputy");
}
public void switchBrand(Brand brand) {
clearBrandStyles();// Help me clear the old, and add the proper styles
}
public void clearBrandStyles() {
for (Brand brand : Brand.values()) {
container.getStyleClass().remove(brand.toString().toLowerCase());
}
}
public void handleBrandSwitch(ActionEvent actionEvent) {
// Get a handle on the calling button
Button btn = (Button) actionEvent.getSource();
// Get access to the brand like Brand.APPLE by name
Brand brand = Brand.valueOf(btn.getText().toUpperCase());
switchBrand(brand);
}
}
/* Brand settings */
.sony {
-fx-background-color: black;
}
.jvc {
-fx-background-color: red;
}
.apple {
-fx-background-color: silver;
}
.coby {
-fx-background-color: gray;
}
package com.example;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception{
Parent root = FXMLLoader.load(getClass().getResource("boombox.fxml"));
primaryStage.setTitle("Boombox");
primaryStage.setScene(new Scene(root, 300, 275));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
<?import javafx.scene.control.Button?>
<?import javafx.scene.layout.HBox?>
<?import javafx.scene.layout.VBox?>
<?import javafx.scene.text.Text?>
<VBox fx:controller="com.example.Controller"
xmlns:fx="http://javafx.com/fxml"
stylesheets="@boombox.css"
fx:id="container">
<HBox>
<Button onAction="#handleBrandSwitch">Sony</Button>
<Button onAction="#handleBrandSwitch">Apple</Button>
<Button onAction="#handleBrandSwitch">JVC</Button>
<Button onAction="#handleBrandSwitch">Coby</Button>
</HBox>
<Text text="Boombox" />
<Button onAction="#handlePlay" text="Play"/>
</VBox>
package com.example.model;
public enum Brand {
JVC("JVC"),
SONY("Sony"),
APPLE("Apple"),
COBY("Coby");
private String mDisplayName;
Brand(String displayName) {
mDisplayName = displayName;
}
public String getDisplayName() {
return mDisplayName;
}
}
2 Answers

Ken Alger
Treehouse TeacherIna;
Great question.
It looks like you have properly cleared the styles already in the switchBrand
method. Therefore we are left with the task of adding the styles for the Brand portion of our task.
If we walk through the steps needed to add a style to our Brand, we probably need to start with getting the current style from our container object, correct? Something like container.getStyleClass()
would be a start then. Now, we want to add a style to that, which we can with the add()
method. To get the brand
information we want, we should be adding brand.toString().toLowerCase()
.
That should get you headed in the right direction, just combine all those together and you should be good. Post back if you are still stuck though.
Happy coding,
Ken
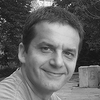
deckey
14,630 PointsHi, you should add styles similarly as they are removed below in the 'clearBrandStyles' method:
But, you should be adding instead of removing and 'Brand' has a 'displayName', instead of toString() method.
Hope it helps a bit, good luck!
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherAs Dejan mentioned, there is indeed a
getDisplayName()
method, which also works for this task.Ken
Inge L
30,058 PointsInge L
30,058 PointsThanks for clearing that up, Ken!
chase singhofen
3,811 Pointschase singhofen
3,811 PointsI dont understand myself.
also, I dont understand how to get back to where i left off at if I sign out and sign back in. i keep having to re-do all the exercises.