Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial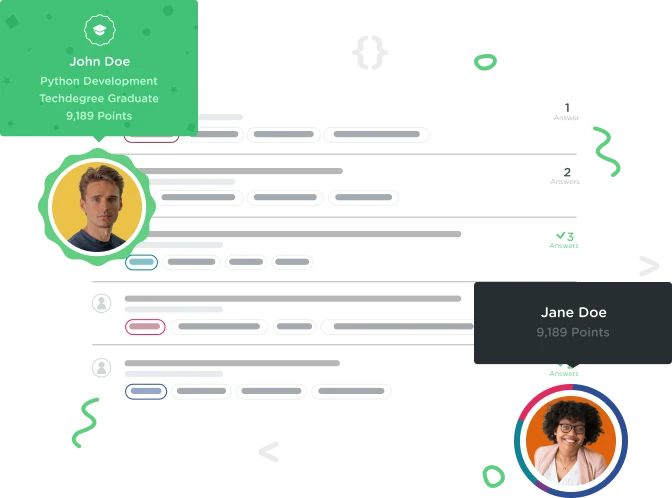

Lewis Mrozek
1,538 PointsHow does the self.use_gas() work?
def go(self, speed):
if self.use_gas():
if not self.is_moving:
print('The car starts moving')
self.is_moving = True
print(f'The car is going {speed}.')
else:
print('You are out of gas.')
self.stop()
def use_gas(self):
self.gas -= 50
if self.gas <= 0:
return False
else:
return True
When we call the "if self.use_gas()", does the call make the use.gas program fully run? It kind of makes sense, but I'm not 100% fully understanding how it's putting it all together. Can someone expand on the concept a bit?
1 Answer
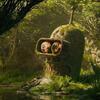
Samuel Tredgett
11,372 PointsSo the thing to understand with the use of "self" in python is it is essentially a reference to the structure of the object which is using it.
In the example you've shown, the "self" is the car. So when we say
self.use_gas()
we're saying
car.use_gas()
by proxy, since the "self" in this case is the car.
What's important to understand about this is it's a mechanism of the concept of object oriented programming. The self/car being the object, and use_gas() being the method called on the object.
We can look at this through another example. If we were to have an lamp object, that lamp might have some the function it can use on itself called "light_up".
But we could have many types of lamp, small ones, ones that hang from ceilings, ones that are mounted on walls, etc.
What we'd want is for all of these lamps to have the common ability to use our "light_up" function. So we'd create an object oriented structure in which lamps can have some variation in distinct features, but all access the "light_up" function through the generic structure of the object.
This is where the keyword self comes in. We can use this generic structure to reference the object loosely and allow all those unique variations of the lamp to use this same function. This is the same as in the instance of your car. In the code below you'll see I define a light_up function for some lamp class objects that will change a boolean value for that object, and print some text referencing the objects name.
def light_up(self):
self.light_on = True
print(f"The {self.size} {self.colour} lamp was turned on!")
The result of this would be best highlighted by showing how it would compile in two different instances. To do this, we'll now construct the lamp class and generate a few instances to see how they would interact.
class Lamp:
def __init__(self, size, colour, light_on = False):
# Notice how the constructor here defaults our light_on variable to False
self.colour = colour
self.size = size
self.light_on = light_on
#Our light_up function would exist inside the class, as it is a part of the object structure
def light_up(self):
self.light_on = True
print(f"The {self.size} {self.colour} lamp was turned on!")
# Now that we are outside of the close due to our indentation, we can create some examples and test them.
bedside_lamp = Lamp("medium", "cream")
desk_lamp = Lamp("Large", "White")
# The above two lines have now constructed two objects of our Lamp class
# From here we can finally use our methods
bedside_lamp.light_up() # Notice the keyword self does not need to be mentioned in the function call
desk_lamp.light_up()
From the above code, we create our Lamp class, give it some attributes colour and size and light_on. We then are able to use a function assigned to the object. The resultant output to the console (assuming I haven't made any errors in this) would be:
The medium cream lamp was turned on!
The Large White lamp was turned on!
I hope this clears up some of the confusion regarding how objects work. If you'd like anything clarified feel free to respond.
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsGreat answer Samuel Tredgett! Your comment has been moved to answer.